We can insert multiple checkbox values in a single column in the database in PHP with the help of an implode function in the form of an array.
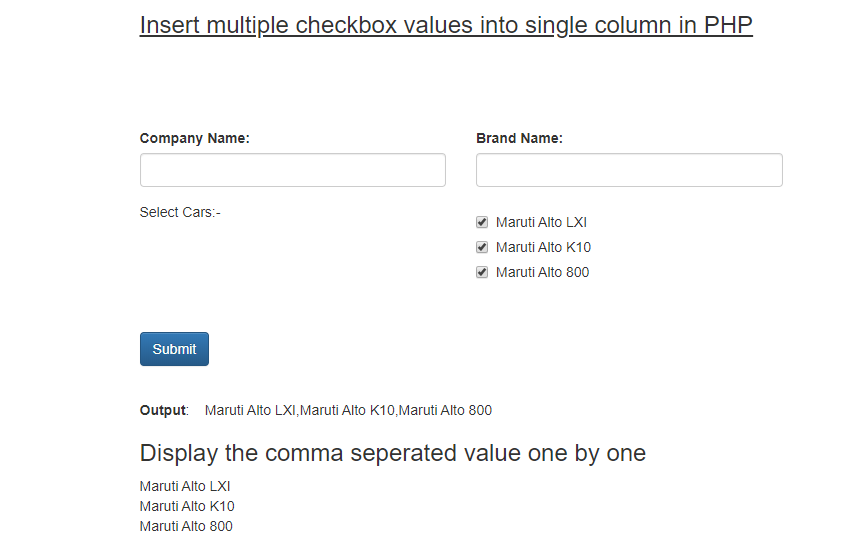
How to insert checkbox array value in the database in PHP
There are two ways we can insert multiple checkbox values in the database.
- We can insert the checkbox values in the form of an array with the help of implode function.
- We can insert the checkbox values in the form of multiple rows one by one in the database.
But in this tutorial, we will use the implode function. You can create a table in your existing database. Here, I am using a table named ‘cars’ that consists of four fields:–id,company_name, brand_name, and car_name.
DDL information of the table
————————————
CREATE TABLE `cars` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT, `company_name` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL, `brand_name` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL,`car_name` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL,PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8mb4
COLLATE=utf8mb4_unicode_ci
connect.php:-
<?php
$servername='localhost';
$username="root";
$password="";
try
{
$con=new PDO("mysql:host=$servername;dbname=database name",$username,$password);
$con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
//echo 'connected';
}
catch(PDOException $e)
{
echo '<br>'.$e->getMessage();
}
?>
<?php
include('connect.php');
if(isset($_POST['save'])){
$company_name = $_POST['company_name'];
$brand_name = $_POST['brand_name'];
//insert checkbox values
$cars = implode(',',$_POST['car_name']);
//insert data into database
$sql="INSERT INTO cars(company_name,brand_name,car_name)VALUES('$company_name','$brand_name','$cars')";
$stmt=$con->prepare($sql);
$stmt->execute();
echo '<div class="alert alert-success">Submitted Successfully<button class="close" id="cl" onclick="coseMsg()" >✗</button></div>';
//insertion complete
}
//get the cars
$stmt2 = $con->prepare("select * from cars");
$stmt2->execute();
$cars = $stmt2->fetchAll(PDO::FETCH_ASSOC);
?>
<html>
<head>
<title style="color:#31d818">ajax example</title>
<link rel="stylesheet" href="bootstrap.css" crossorigin="anonymous">
<!-- Optional theme -->
<link rel="stylesheet" href="bootstrap-theme.css" crossorigin="anonymous">
<style>
.container{
width:50%;
height:30%;
padding:20px;
}
</style>
</head>
<body>
<div class="container">
<h3><u>Insert multiple checkbox values into single column in PHP</u></h3>
<br/><br/><br/><br/>
<form action="" method="post">
<div class="row">
<div class="col-sm-6">
<div class="form-group">
<label for="username">Company Name:</label>
<input type="text" class="form-control" name="company_name">
</div>
</div>
<div class="col-sm-6">
<div class="form-group">
<label for="phone_no">Brand Name:</label>
<input type="text" class="form-control" name="brand_name">
</div>
</div>
</div>
<div class="row">
<div class="col-sm-6">
Select Cars:-
</div>
<div class="col-sm-6">
<div class="checkbox">
<label><input type="checkbox" required="" name="car_name[]" value="Maruti Alto LXI">Maruti Alto LXI</label>
</div>
<div class="checkbox">
<label><input type="checkbox" required="" name="car_name[]" value="Maruti Alto K10">Maruti Alto K10</label>
</div>
<div class="checkbox">
<label><input type="checkbox" required="" name="car_name[]" value="Maruti Alto 800">Maruti Alto 800</label>
</div>
</div>
</div>
<br/><br/>
<button type="submit" class="btn btn-primary" name="save">Submit</button>
</form>
<br/>
<?php
foreach($cars as $car){
?>
<b>Output</b>: <?php echo $car['car_name'];?>
<?php
}
?>
<br/>
<!--To display the comma seperated value from database one by one-->
<h3>Display the comma seperated value one by one</h3>
<?php
foreach($cars as $car){
$cars = explode(',',$car['car_name']);
for($i=0;$i<sizeof($cars);$i++){
echo $cars[$i].'<br>';
}
}
?>
</div>
<script src="jquery-3.2.1.min.js"></script>
<script src="bootstrap.min.js"></script>
<script>
function closeMsg()
{
$('#cl').hide();
}
</script>
</body>
</html>
NOTE*
——–
Download the bootstrap CSS and js files from google and include the path of the files in the href attribute of link tag and src attribute of the script tag respectively.
CONCLUSION:- I hope this article will help you to understand. If you have any doubt then please leave your comment below.
You ought to be a part of a contest for one of the highest quality sites on the net.
I will recommend this website!
Greate pieces. Keep posting such kind of info on your page.
Im really impressed by your site.
Hello there, You’ve performed a fantastic job. I’ll
definitely digg it and individually recommend to my friends.
I’m confident they will be benefited from this website.
This design is wicked! You obviously know how to keep a reader entertained.
Between your wit and your videos, I was almost moved to start my own blog (well,
almost…HaHa!) Fantastic job. I really loved what you had to say, and more than that, how you presented it.
Too cool!