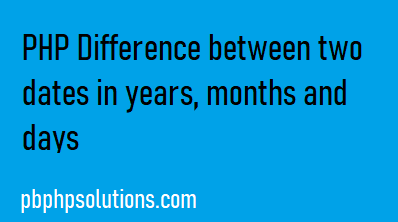
To calculate the PHP difference between two dates in years, months, and days, we have to use three functions
- strtotime() function
- floor() function()
- abs() function
Read Here, strtotime, floor and abs functions in PHP
Let us consider two dates and store in variables.
$date1 = ‘2016-06-01’;
$date2 = ‘2020-08-08’;
Now, the difference between these two dates will be
$diff = abs(strtotime($date2)-strtotime($date1));
To calculate the year, divide the difference by total seconds in the year that is 365*60*60*24
Now, the year between these two dates will be
$years = floor($diff / (365*60*60*24));
To calculate the month, subtract the year from the date difference and divide the result by total seconds in a month that is 30*60*60*24
Now, the month between these two dates will be
$months = floor(($diff - $years * 365*60*60*24) / (30*60*60*24));
To calculate the day, subtract the date difference from the year and month and divide the result by total seconds in a day that is 60*60*24
Now, the days between these two dates will be
$days = floor(($diff - $years * 365*60*60*24 - $months*30*60*60*24)/ (60*60*24));
Complete Code:-
<?php
$date1 = '2016-06-01';
$date2 = '2020-08-08';
$diff = abs(strtotime($date2)-strtotime($date1));
$years = floor($diff / (365*60*60*24));
$months = floor(($diff - $years * 365*60*60*24) / (30*60*60*24));
$days = floor(($diff - $years * 365*60*60*24 - $months*30*60*60*24)/ (60*60*24));
echo 'The year between these two dates is '.$years.' Years'.'<br>';
echo 'The month between these two dates is '.$months.' Month'.'<br>';
echo 'The days between these two dates is '.$days.' Days'.'<br>';
?>
How to calculate no of days between two dates in PHP
<?php
$date1 = '2016-06-01';
$date2 = '2020-08-08';
$diff = abs(strtotime($date2)-strtotime($date1));
$days = $diff/(60*60*24);
echo 'The total no of days between these two dates: $days;
?>
Conclusion:- I hope this tutorial will help you to understand the difference between two dates in PHP in years, months, and days.
What a information of un-ambiguity and preserveness of valuable familiarity regarding unpredicted
feelings.
Hi there to every body, it’s my first go to see of
this webpage; this weblog carries amazing and in fact good stuff designed for readers.