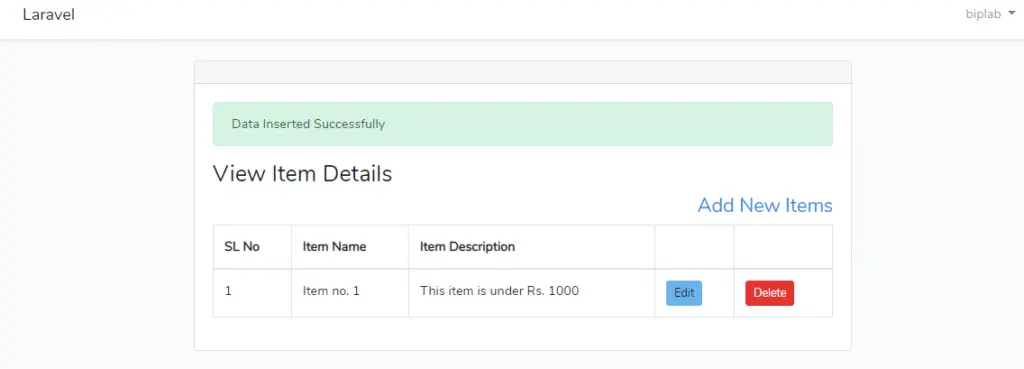
Hi friends, before getting started with the tutorial CRUD operation in laravel 5.8, I want to say that Laravel latest version has changed a little bit process of coding. Assuming that you already have installed Laravel 5.8 in your system. If not yet installed then also read, how to create laravel project from scratch for beginners.
The requirements for the CRUD operation in Laravel 5.8 are as follows-
- We need a table in the database.
- Route Configuration in web.php file.
- A controller containing all the required functions.
- A model containing all the fields of the table in the database.
- We need a folder containing the following files in the resources/views folder of the public HTML folder. I have a folder name item.
1. add.blade.php
2. edit.blade.php
3. view_item_details.blade.php
All the urls are processed through web.php file inside the routes folder.
Let’s start the operation in detail
To set up the database configuration go to .env file in your laravel project and set your database as follows
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=root
DB_PASSWORD=
Now, create a table directly in the PHPMyAdmin of the local server. In my case (WAMP Server).
DDL information of the table
CREATE TABLE items
(id
int(10) unsigned NOT NULL AUTO_INCREMENT,item_name
varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,item_description
varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,created_at
timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp(),updated_at
timestamp NOT NULL DEFAULT ‘0000-00-00 00:00:00’,
PRIMARY KEY (id
)
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
Now, we will set up all the route connections for the URL’s including create, read, edit, update and delete in the web.php file inside the routes folder.
web.php
<?php
use Illuminate\Support\Facades\Route;
/*
|--------------------------------------------------------------------------
| Web Routes
|--------------------------------------------------------------------------
|
| Here is where you can register web routes for your application. These
| routes are loaded by the RouteServiceProvider within a group which
| contains the "web" middleware group. Now create something great!
|
*/
Route::get('/', function () {
return view('welcome');
});
Auth::routes();
Route::get('/home', [App\Http\Controllers\HomeController::class, 'index'])->name('home');
Route::get('/items',[App\Http\Controllers\ItemController::class, 'index']);
Route::get('/additem',[App\Http\Controllers\ItemController::class, 'create']);
Route::post('/submititem',[App\Http\Controllers\ItemController::class, 'store']);
Route::get('/edit/{id}',[App\Http\Controllers\ItemController::class, 'edit']);
Route::post('/update/{id}',[App\Http\Controllers\ItemController::class, 'update']);
Route::get('/delete/{id}',[App\Http\Controllers\ItemController::class, 'destroy']);
Now, open your command prompt terminal and go to your project folder inside the root directory of your local server and create the controller using the below command
php artisan make:controller ItemController
ItemController
<?php
namespace App\Http\Controllers;
//use HasFactory;
use Illuminate\Http\Request;
use App\Models\Item;
class ItemController extends Controller
{
//
public function index(){
$items = Item::all();
return view('item.view_item_details',compact('items'));
}
public function create(){
return view('item.add');
}
public function store(Request $request){
if($request->isMethod('POST'))
{
$request->validate([
'item_name'=> 'required',
'item_description'=>'required',
]);
$data = new Item;
$data->item_name = $request->item_name;
$data->item_description = $request->item_description;
$data->save();
return redirect('/items')->with('success','Data Inserted Successfully');
}
}
public function edit(Request $request, $id){
$edititem = Item::findOrFail($id);
return view('item.edit',compact('edititem','id'));
}
public function update(Request $request, $id)
{
if($request->isMethod('POST'))
{
$request->validate([
'item_name'=> 'required',
'item_description'=>'required',
]);
$data = Item::findOrFail($id);
$data->item_name = $request->item_name;
$data->item_description= $request->item_description;
$data->created_at = now();
$data->updated_at = now();
$data->save();
return redirect('/items')->with('success','Data Updated Successfully');
}
}
public function destroy($id){
$deleteitems = Item::findOrFail($id);
$deleteitems->delete();
return redirect('/items')->with('danger','Data deleted Successfully');
}
}
Now, after the successful creation of the controller,create a model using the below command
php artisan make:model Item
Item.php (Model)
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Item extends Model
{
use HasFactory;
protected $table = 'items';
protected $fillable = [
'item_name','item_description',
];
}
Now, we will create the blade files which are shown in the browser for the CRUD operation. Blade files are given below one by one.
view_item_details.blade.php (Here you will see all the items inserted)
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header"></div>
<div class="card-body">
@if (session('success'))
<div class="alert alert-success" role="alert">
{{ session('success') }}
</div>
@endif
<h3>View Item Details</h3>
<h4 style="text-align: right;"><a href="/additem">Add New Items</a></h4>
<table class="table table-bordered">
<thead>
<tr>
<th>SL No</th>
<th>Item Name</th>
<th>Item Description</th>
<th></th>
<th></th>
</tr>
</thead>
<tbody>
@foreach($items as $key=>$value)
<tr>
<td>{{$key+1}}</td>
<td>{{$value->item_name}}</td>
<td>{{$value->item_description}}</td>
<td>
<a href="/edit/{{$value->id}}" class="btn btn-info btn-sm">Edit</a>
</td>
<td>
<a href="/delete/{{$value->id}}" class="btn btn-danger btn-sm" onclick="return confirm('Are you sure?')">Delete</a>
</td>
</tr>
@endforeach
</tbody>
</table>
</div>
</div>
</div>
</div>
</div>
@endsection
add.blade.php ( Here you will insert the information)
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header"></div>
<div class="card-body">
@if (session('status'))
<div class="alert alert-success" role="alert">
{{ session('status') }}
</div>
@endif
<h3>Insert Item Details</h3>
<form method="POST" action="/submititem">
{{csrf_field()}}
<div class="form-group row">
<label for="item_name" class="col-md-4 col-form-label text-md-right">{{ __('Item Name') }}</label>
<div class="col-md-6">
<input id="item_name" type="text" class="form-control @error('item_name') is-invalid @enderror" name="item_name" value="{{ old('item_name') }}" required autocomplete="name" autofocus>
@error('item_name')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row">
<label for="item_description" class="col-md-4 col-form-label text-md-right">{{ __('Item Description') }}</label>
<div class="col-md-6">
<input id="item_description" type="text" class="form-control @error('item_description') is-invalid @enderror" name="item_description" value="{{ old('item_description') }}" required autocomplete="item_description">
@error('item_description')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row mb-0">
<div class="col-md-6 offset-md-4">
<button type="submit" class="btn btn-primary">
{{ __('Add') }}
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
@endsection
edit.blade.php ( Here you will edit the required information)
@extends('layouts.app')
@section('content')
<div class="container">
<div class="row justify-content-center">
<div class="col-md-8">
<div class="card">
<div class="card-header"></div>
<div class="card-body">
@if (session('status'))
<div class="alert alert-success" role="alert">
{{ session('status') }}
</div>
@endif
<h3>Insert Item Details</h3>
<form method="POST" action="/update/{{$id}}">
{{csrf_field()}}
<div class="form-group row">
<label for="item_name" class="col-md-4 col-form-label text-md-right">{{ __('Item Name') }}</label>
<div class="col-md-6">
<input id="item_name" type="text" class="form-control @error('item_name') is-invalid @enderror" name="item_name" value="{{ $edititem->item_name }}" required autocomplete="name" autofocus>
@error('item_name')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row">
<label for="item_description" class="col-md-4 col-form-label text-md-right">{{ __('Item Description') }}</label>
<div class="col-md-6">
<input id="item_description" type="text" class="form-control @error('item_description') is-invalid @enderror" name="item_description" value="{{ $edititem->item_description }}" required autocomplete="item_description">
@error('item_description')
<span class="invalid-feedback" role="alert">
<strong>{{ $message }}</strong>
</span>
@enderror
</div>
</div>
<div class="form-group row mb-0">
<div class="col-md-6 offset-md-4">
<button type="submit" class="btn btn-primary">
{{ __('Update') }}
</button>
</div>
</div>
</form>
</div>
</div>
</div>
</div>
</div>
@endsection
To delete, you can check the destroy function inside the controller to know how it works.
Conclusion:- I hope this article will help you to understand the basic overview of the CRUD operation in Laravel 5.8
Also, Read How To Run Laravel On Localhost Without PHP Artisan Serve
If you want to know more about laravel topics, you can read this article
Thanks a lot for the blog article. Really thank you! Awesome. Gabriel Any Yeorgi
Hi there colleagues, its fantastic piece of writing about tutoringand fully defined, keep it up all the time. Tiffani Ingmar Taylor
Thank you for reading my article
I think this is one of the so much significant information for me.
And i am satisfied reading your article. However should commentary on few basic
issues, The web site taste is wonderful, the articles is really nice :
D. Just right activity, cheers 0mniartist asmr
Somebody essentially help to make critically posts I
might state. That is the first time I frequented your web page
and up to now? I amazed with the research you made to create this particular submit amazing.
Magnificent activity! 0mniartist asmr
Have you ever thought about writing an e-book or guest authoring on other websites?
I have a blog centered on the same information you discuss and would
love to have you share some stories/information. I
know my viewers would appreciate your work. If you are even remotely interested, feel free to send me
an e-mail. asmr 0mniartist
I read this article fully about the resemblance of hottest and previous technologies, it’s remarkable article.
A person necessarily lend a hand to make critically articles I would state.
This is the first time I frequented your web page and so far?
I amazed with the analysis you made to create this actual publish incredible.
Magnificent job!
This is very interesting, You are a very skilled blogger. I’ve joined your feed
and look forward to seeking more of your excellent post.
Also, I have shared your website in my social networks!
When someone writes an piece of writing he/she keeps the idea of a user in his/her brain that how a user can know it.
So that’s why this piece of writing is amazing.
Thanks!
Hey there would you mind letting me know which hosting company you’re using?
I’ve loaded your blog in 3 different web browsers
and I must say this blog loads a lot quicker then most.
Can you suggest a good web hosting provider at a fair
price? Thanks, I appreciate it!
Hey there, thanks for your observation. I am using Hostinger for my blog but if you want to buy Linux shared hosting for web development then I would recommend Hostgator. If you are interested in cheap and reliable web hosting based on speed and performance then go through this link. https://hostgator-india.sjv.io/J3krE
If you want to buy hosting for blogging at a fair price then go with hostinger.
Do you have a spam issue on this site; I also am a blogger, and I was curious about your situation; many of
us have created some nice methods and we are looking to exchange methods with others, be sure to shoot me an e-mail if interested.
Oh my goodness! Amazing article dude! Thank you, However I
am having troubles with your RSS. I don’t understand why I am unable to join it.
Is there anyone else having identical RSS issues? Anybody who knows
the solution will you kindly respond? Thanx!!
What’s up to every single one, it’s in fact a good for me to go to see this web page, it includes
useful Information.
This blog was… how do I say it? Relevant!! Finally I’ve found something that helped me.
Many thanks!
Nice post. I was checking continuously this blog and I am impressed!
Very useful information specifically the last part :
) I care for such info a lot. I was seeking this particular info for
a very long time. Thank you and best of luck.
I have read so many posts regarding the blogger lovers except this piece of writing
is actually a good piece of writing, keep it up.
Ahaa, its good discussion about this piece of writing at this
place at this website, I have read all that, so now me also
commenting at this place.
It is the best time to make a few plans for the long run and it’s time to be happy.
I have read this publish and if I may I desire to suggest you few fascinating things or suggestions.
Maybe you could write subsequent articles regarding this
article. I wish to read more things about it!
I’m extremely inspired together with your writing
talents and also with the structure for your weblog.
Is that this a paid theme or did you modify
it yourself? Anyway stay up the nice high quality writing, it’s rare to look a nice weblog like this one nowadays..
This design is incredible! You most certainly know how to
keep a reader amused. Between your wit and your videos, I was almost
moved to start my own blog (well, almost…HaHa!) Great job.
I really enjoyed what you had to say, and more than that, how you presented it.
Too cool!
Wow, incredible blog layout! How long have you been blogging for?
you made blogging look easy. The overall look of your website is excellent, as well as the content!
I am really enjoying the theme/design of your website.
Do you ever run into any internet browser compatibility problems?
A number of my blog visitors have complained about my blog not operating
correctly in Explorer but looks great in Safari. Do you have any advice to help fix this issue?
Does your website have a contact page? I’m having trouble locating it but,
I’d like to shoot you an email. I’ve got some suggestions for your blog you might be interested in hearing.
Either way, great blog and I look forward to seeing it
expand over time.
I do believe all of the concepts you have presented on your
post. They’re very convincing and can definitely work.
Nonetheless, the posts are very short for newbies.
May just you please lengthen them a little from next time?
Thanks for the post.
I constantly spent my half an hour to read this web site’s content everyday along with a mug of coffee.
I think the admin of this website is really working hard for his site, for the reason that here every material is quality based material.
Hi my friend! I wish to say that this post is awesome, nice written and include almost all significant infos. I would like to see more posts like this.