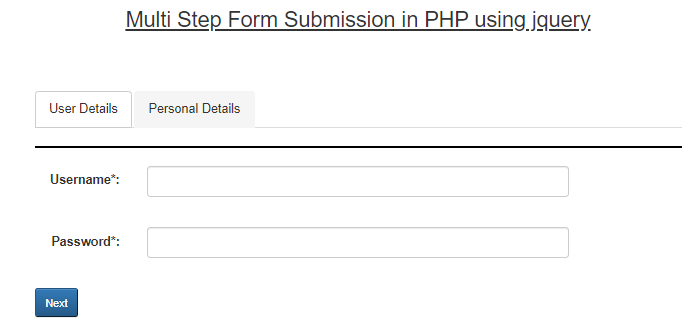
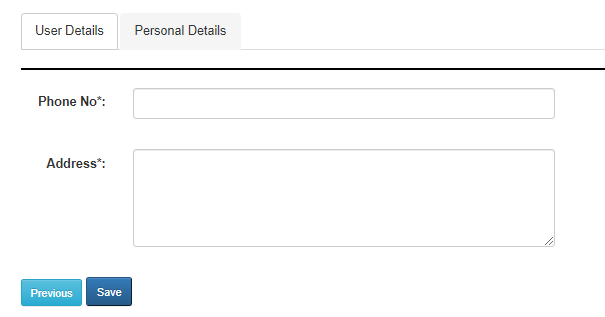
Hi Friends, In this tutorial, we will learn how to create multi-step form submission in PHP using jQuery. It is very simple and in order to do this, please follow the below steps one by one.
- We have to create a table in our database.
I have a table name “stepformtbl” in my database.
DDL information of the table
CREATE TABLE stepformtbl
(id
int(10) unsigned NOT NULL AUTO_INCREMENT,username
varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,password
varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,phone_no
varchar(20) COLLATE utf8mb4_unicode_ci DEFAULT NULL,address
varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,created_at
timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp(),updated_at
timestamp NOT NULL DEFAULT ‘0000-00-00 00:00:00’,
PRIMARY KEY (id
)
) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
2. Create the input fields in HTML using active tabs and inactive tabs to identify the input fields according to the tabs. We will do this with the help of jQuery. In the HTML file, the id attribute of each input field is very important.
By using the id of input fields, we will do the validation part. Please follow the below HTML code to understand how the input fields are changing according to the navigation tabs.
HTML Code:-
<div class="container">
<h3 align="center"><u>Multi Step Form Submission in PHP using jquery </u></h3>
<br/><br/><br/>
<ul class="nav nav-tabs">
<li class="nav-item">
<a class="nav-link active_tab1" style="border:1px solid #ccc;font-size: 14px;background-color: #fff;color: #333" id="list_user_details">User Details</a>
</li>
<li class="nav-item">
<a class="nav-link inactive_tab1" id="list_personal_details" style="background-color: #f5f5f5;color: #333;font-size: 14px;">Personal Details</a>
</li>
</ul>
<hr style="border: 1px solid black;">
<form class="form-horizontal" action="" method="post">
<div class="tab-content">
<div class="tab-pane active" id="user_details">
<div class="form-group">
<label class="control-label col-sm-2" for="Username">Username*:</label>
<div class="col-sm-8">
<input type="text" name="username" id="user" class="form-control form-control-sm">
</div>
</div>
<br/>
<div class="form-group">
<label class="control-label col-sm-2" for="Password">Password*:</label>
<div class="col-sm-8">
<input type="password" id="pass" name="password" class="form-control form-control-sm">
</div>
</div>
<br>
<button type="button" class="btn-sm btn-primary" id="btn_us">Next</button>
</div>
<div class="tab-pane" id="personal_details">
<div class="form-group">
<label class="control-label col-sm-2" for="Phone No">Phone No*:</label>
<div class="col-sm-8">
<input type="text" name="phone_no" class="form-control form-control-sm">
</div>
</div>
<br/>
<div class="form-group">
<label class="control-label col-sm-2" for="Address">Address*:</label>
<div class="col-sm-8">
<textarea name="address" class="form-control" rows="5"></textarea>
</div>
</div>
<br>
<button type="button" name="previous_btn_personal_details" id="previous_btn_personal_details" class="btn btn-info btn-sm">Previous</button>
<button type="submit" class="btn-sm btn-primary" name="save">Save</button>
</div>
</div>
</form>
</div>
If you look at the HTML code, I have created two navigation tabs with class names “active tab1” and “inactive tab1” with the id attribute of each inside the hyperlink tag.
Also read, Insert Multiple Rows into MySQL with PHP Using Foreach Arrays
jQuery multi-step form submission example
3. Validate the input fields using jQuery to proceed to the next step. If you do not enter the information in the first step or in the previous step then you can not proceed to the next step. This is done by only Jquery.
<script src="jquery-3.2.1.min.js"></script>
<script src="bootstrap.min.js"></script>
<script>
//step form validation
$('#btn_us').click(function(){
//alert('ok');
var user = $('#user').val();
var password = $('#pass').val();
if(user==''){
alert('Please enter the username');
}
if(password==''){
alert('Enter the password');
}
else{
$('#list_user_details').removeClass('active active_tab1');
$('#list_user_details').removeAttr('href data-toggle');
$('#user_details').removeClass('active');
$('#list_user_details').addClass('inactive_tab1');
$('#list_personal_details').removeClass('inactive_tab1');
$('#list_personal_details').addClass('active_tab1 active');
$('#list_personal_details').attr('href', '#personal_details');
$('#list_personal_details').attr('data-toggle', 'tab');
$('#personal_details').addClass('active in');
}
});
$('#previous_btn_personal_details').click(function(){
$('#list_personal_details').removeClass('active active_tab1');
$('#list_personal_details').removeAttr('href data-toggle');
$('#personal_details').removeClass('active');
$('#list_personal_details').addClass('inactive_tab1');
$('#list_user_details').removeClass('inactive_tab1');
$('#list_user_details').addClass('active_tab1 active');
$('#list_user_details').attr('href', '#personal_details');
$('#list_user_details').attr('data-toggle', 'tab');
$('#user_details').addClass('active in');
});
if you look at the above code validation starts with the if else condition. If information is empty then it will ask you to enter the required information. If it is ok then it will move to the else condition.
Steps while clicking on the NEXT button
When we click on the NEXT button, the following classes of jquery are applied.
- removeClass().
- addClass().
- removeAttr().
- attr().
- data-toggle property
data-toggle is basically used to jump from one div to another div.
If you want to know these classes in detail then follow this link Jquery classes with examples.
So, after clicking on the next button, we will remove the section of the active tab with the help of removeClass().
We will add the section of inactive tab with the help of addClass().
We will get all the attributes, input fields, and id of the personal details tab using the attr() method of Jquery.
4. If the validation is ok then it is time to submit the data to the database. We will do this with help of PHP.
<?php
include('connect.php');
if(isset($_POST['save']))
{
$username = $_POST['username'];
$password = $_POST['password'];
$phone_no = $_POST['phone_no'];
$address = $_POST['address'];
//echo $username.$password.$phone_no.$address;
//insert into the table in the database
$sql="INSERT INTO stepformtbl(username,password,phone_no,address)VALUES('$username','$password','$phone_no','$address')";
$stmt=$con->prepare($sql);
$stmt->execute();
echo "<script type='text/javascript'>";
echo "alert('Submitted successfully')";
echo "</script>";
}
?>
Complete Code:-
<?php
include('connect.php');
if(isset($_POST['save']))
{
$username = $_POST['username'];
$password = $_POST['password'];
$phone_no = $_POST['phone_no'];
$address = $_POST['address'];
//echo $username.$password.$phone_no.$address;
//insert into the table in the database
$sql="INSERT INTO stepformtbl(username,password,phone_no,address)VALUES('$username','$password','$phone_no','$address')";
$stmt=$con->prepare($sql);
$stmt->execute();
echo "<script type='text/javascript'>";
echo "alert('Submitted successfully')";
echo "</script>";
}
?>
<html>
<head>
<title>ajax example</title>
<link rel="stylesheet" href="bootstrap.css" crossorigin="anonymous">
<!-- Optional theme -->
<link rel="stylesheet" href="bootstrap-theme.css" crossorigin="anonymous">
<style>
.container{
width:50%;
height:30%;
padding:20px;
}
</style>
</head>
<body>
<div class="container">
<h3 align="center"><u>Multi Step Form Submission in PHP using jquery </u></h3>
<br/><br/><br/>
<ul class="nav nav-tabs">
<li class="nav-item">
<a class="nav-link active_tab1" style="border:1px solid #ccc;font-size: 14px;background-color: #fff;color: #333" id="list_user_details">User Details</a>
</li>
<li class="nav-item">
<a class="nav-link inactive_tab1" id="list_personal_details" style="background-color: #f5f5f5;color: #333;font-size: 14px;">Personal Details</a>
</li>
</ul>
<hr style="border: 1px solid black;">
<form class="form-horizontal" action="" method="post">
<div class="tab-content">
<div class="tab-pane active" id="user_details">
<div class="form-group">
<label class="control-label col-sm-2" for="Username">Username*:</label>
<div class="col-sm-8">
<input type="text" name="username" id="user" class="form-control form-control-sm">
</div>
</div>
<br/>
<div class="form-group">
<label class="control-label col-sm-2" for="Password">Password*:</label>
<div class="col-sm-8">
<input type="password" id="pass" name="password" class="form-control form-control-sm">
</div>
</div>
<br>
<button type="button" class="btn-sm btn-primary" id="btn_us">Next</button>
</div>
<div class="tab-pane" id="personal_details">
<div class="form-group">
<label class="control-label col-sm-2" for="Phone No">Phone No*:</label>
<div class="col-sm-8">
<input type="text" name="phone_no" class="form-control form-control-sm">
</div>
</div>
<br/>
<div class="form-group">
<label class="control-label col-sm-2" for="Address">Address*:</label>
<div class="col-sm-8">
<textarea name="address" class="form-control" rows="5"></textarea>
</div>
</div>
<br>
<button type="button" name="previous_btn_personal_details" id="previous_btn_personal_details" class="btn btn-info btn-sm">Previous</button>
<button type="submit" class="btn-sm btn-primary" name="save">Save</button>
</div>
</div>
</form>
</div>
<script src="jquery-3.2.1.min.js"></script>
<script src="bootstrap.min.js"></script>
<script>
//step form validation
$('#btn_us').click(function(){
//alert('ok');
var user = $('#user').val();
var password = $('#pass').val();
if(user==''){
alert('Please enter the username');
}
if(password==''){
alert('Enter the password');
}
else{
$('#list_user_details').removeClass('active active_tab1');
$('#list_user_details').removeAttr('href data-toggle');
$('#user_details').removeClass('active');
$('#list_user_details').addClass('inactive_tab1');
$('#list_personal_details').removeClass('inactive_tab1');
$('#list_personal_details').addClass('active_tab1 active');
$('#list_personal_details').attr('href', '#personal_details');
$('#list_personal_details').attr('data-toggle', 'tab');
$('#personal_details').addClass('active in');
}
});
$('#previous_btn_personal_details').click(function(){
$('#list_personal_details').removeClass('active active_tab1');
$('#list_personal_details').removeAttr('href data-toggle');
$('#personal_details').removeClass('active');
$('#list_personal_details').addClass('inactive_tab1');
$('#list_user_details').removeClass('inactive_tab1');
$('#list_user_details').addClass('active_tab1 active');
$('#list_user_details').attr('href', '#personal_details');
$('#list_user_details').attr('data-toggle', 'tab');
$('#user_details').addClass('active in');
});
</script>
</body>
</html>
NOTE*
Download the bootstrap css and js files from google and include the path of the files in the href attribute of link tag and src attribute of the script tag respectively.
Also Read, CRUD Operation In Laravel 5.8 Step By Step For Beginners
CONCLUSION:- I hope this article will help you to create multi-step form submission in PHP using jQuery. If you have any doubt then please leave your comment below.
Hi there, I would like to subscribe for this webpage to obtain most
recent updates, so where can i do it please help out.
Hi there friends, fastidious piece of writing and nice urging commented at
this place, I am truly enjoying by these.
Excellent post. I used to be checking continuously this weblog and I am impressed!
Very useful information specially the closing part
🙂 I care for such info a lot. I was seeking this certain info for a very long time.
Thanks and good luck.