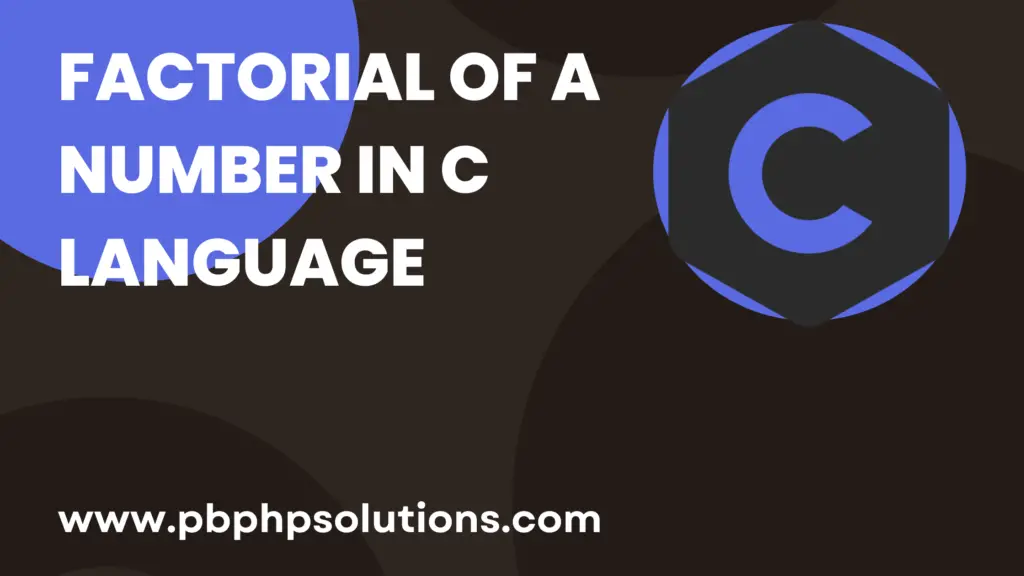
In this tutorial, you will learn to write a C program to find factorial of a number in the C language with example. I will explain the step-by-step process to find the factorial of any given number.
Also read, For Loop Statement in C With Example
Logic to write C program to find factorial of a number
Step 1:- Initially, we will declare three integer numbers as shown below
int i, n, fact;
whereas ‘i’ is used as the increment when the for loop executes
‘n’ is considered as the number for which factorial is defined
‘fact’ is the variable used to store the result of the factorial.
Step 2:- Next, we will run a for loop up to the given factorial number. Suppose, we run a for loop from ‘1’ to ‘5’ for the number 5 as shown below
1
2
3
4
5
Step 3:- Now, we will declare an integer variable ‘fact’ and assign the value 1 as shown below
fact = 1;
Step 4:- Now, each incremented value of the for loop will be multiplied by the above fact variable, and also the result will be stored in the ‘fact’ variable one by one as shown below
For '1', the value of the fact variable will be fact = fact*1 i.e. fact = 1*1 and it becomes face = 1;
For '2', the value of the fact variable will be fact = fact*2 i.e. fact = 1*2 and it becomes fact = 2;
For '3', the value of the fact variable will be fact = fact*3 i.e. fact = 2*3 and it becomes fact = 6;
For '4', the value of the fact variable will be fact = fact*4 i.e. fact = 6*4 and it becomes fact = 24;
For '5', the value of the fact variable will be fact = fact*5 i.e. fact = 24*5 and it becomes fact = 120;
So, the final factorial value of the number 5 is 120
Complete Code:-
#include <stdio.h>
main()
{
int i, n, fact;
printf("\n Enter the number : ");
scanf("%d",&n);
fact = 1;
//Loop to generate the factorial number
for(i=1;i<=n;i++)
{
fact = fact*i;
}
printf("\n The factorial of given number is: %d",fact);
}
After running the above program, the user has to enter the number manually for which the factorial is generated and press the ENTER key. Now, the program will be executed and the result will be displayed as shown below
Enter the number : 5
The factorial of given number is: 120
Process returned 0 (0x0) execution time : 2.953 s
Press any key to continue.
Conclusion:- I hope this tutorial will help you to find the factorial of any given number easily. If there is any doubt then please leave a comment below.