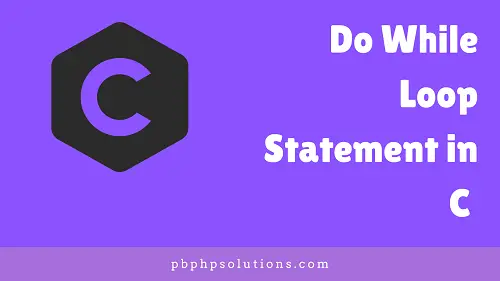
A do-while loop in C is also used to execute and repeat a block of statements depending on a certain condition. It has the following form
do
{
<statement Block>
}
while(<condition>);
where the <condition> is the relational or logical expression that will have the value true or false.
- When the above statement is executed, the computer will execute the statement block without checking the value of the condition.
- After the statement block completes its execution once, the condition is evaluated.
- If the value of the condition is true, the statement block is executed again and is repeated until the condition is false.
Note that the statement block is executed at least once for any value true or false of the condition.
Also read, While Loop in C with Example
Let us take an example below
Print natural numbers from 1 to n using the do while loop in C language
Complete Code:-
#include <stdio.h>
main()
{
int i, n;
printf("Enter the value to n:");
scanf("%d",&n);
//do while loop to print the numbers
i=1;
do
{
i++;
printf("\n%d",i);
}
while(i<=n);
}
After running the above program, the user has to enter the nth value manually and press the ENTER key.
Enter the value to n: 5
Then the program will be executed and the result will be displayed as shown below
2
3
4
5
6
Process returned 0 (0x0) execution time : 1.759 s
Press any key to continue.
Explanation of the output:-
- At first, the initial value of i is incremented once and it becomes 2 i.e. the value of i is now 2.
- Now 2 is compared with 5 and if it is less than 5 then the value of i is incremented and it becomes 3.
- Now 3 is compared with 5 and if it is less than 5 then the value of i is incremented and it becomes 4.
- Now 4 is compared with 5 and if it is less than 5 then the value of i is incremented and it becomes 5.
- Now 5 is compared with 5 and if it is less than or equal to 5 then the value of i is incremented and it becomes 6
Conclusion:- I hope this tutorial will help you understand the concept of Do while loop structure. If there is any doubt then please leave a comment below