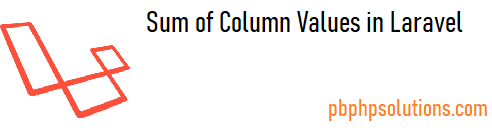
In this tutorial, I will discuss two examples of how to get sum of column values in laravel with the help of laravel query builder. This is very simple and also important if you are working in web development using laravel. Let us take the example one by one below
Also read, How to pass data to all views in laravel
Examples to get sum of column values in laravel
Before getting started with this, we have to create a table in the database. So, let’s create a table
DDL information of the table
CREATE TABLE product_info (
id int(10) NOT NULL AUTO_INCREMENT,
product varchar(255) NOT NULL,
quantity varchar(255) NOT NULL,
price varchar(255) NOT NULL,
created_at timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp(),
updated_at timestamp NOT NULL DEFAULT '0000-00-00 00:00:00',
PRIMARY KEY (id)
) ENGINE=MyISAM AUTO_INCREMENT=4 DEFAULT CHARSET=latin1
Below is the table for your reference
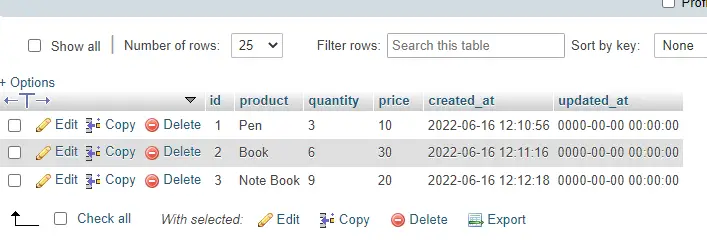
- Using sum() method:- The sum() method returns the sum of a particular column or sum of a particular column with where condition.
Here, we will get the sum of the price column from the table.
Query for sum():-
DB::table('product_info')->sum('price');
Query for sum() with where condition:-
DB::table('product_info')->where('quantity','>',3)->sum('price');
- Using DB::raw() method:- This method also returns the sum of a particular column or multiplication of more than one column or sum of multiple columns. Also, we can evaluate this method with where conditions.
Here, we will get the amount by multiplying the price and quantity column along with where the condition
Query for DB::raw() method:-
$productdata = DB::table('product_info')->select(DB::raw('SUM(quantity*price) as amount'))->get();
foreach($productdata as $product){
$amount = $product->amount;
}
Query for DB::raw() with where condition
//amount with where condition
$productdata_using_where = DB::table('product_info')->select(DB::raw('SUM(quantity*price) as amount'))->where('quantity','>',3)->get();
foreach($productdata_using_where as $value){
$amount_using_where = $value->amount;
}
Conclusion:- I hope this tutorial will help you to understand the overview. If there is any doubt then please leave a comment below