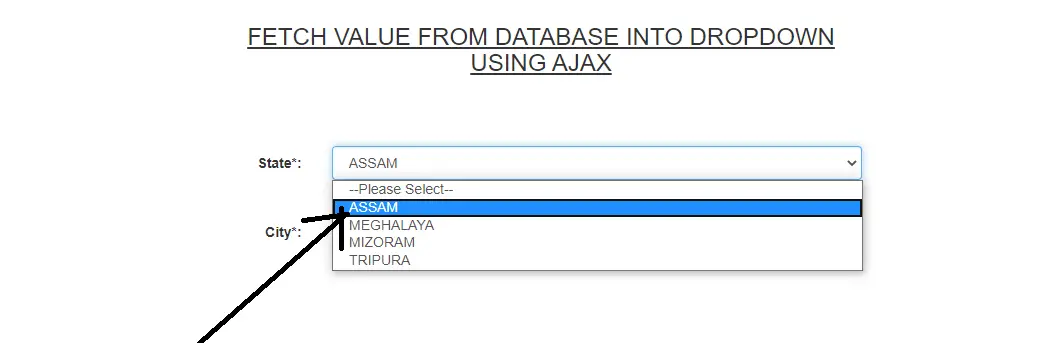
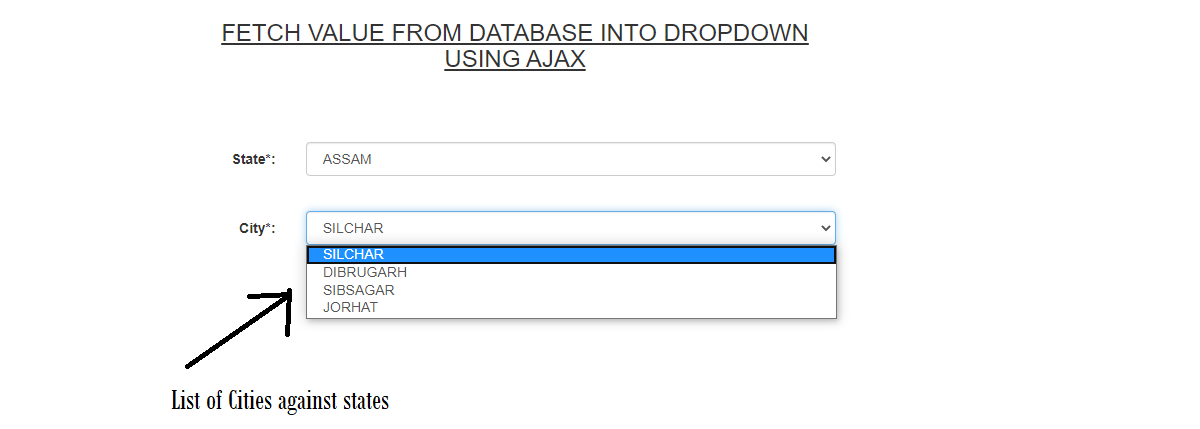
In this tutorial, I am going to explain how to append data to dropdown list using jquery ajax PHP. In order to do this, we use onchange function of jquery and send the data with get() or post() method to the ajax request and take the response data using ‘JSON’.
For eg:- We have a table name ‘state’ and another table name ‘city’. Now, when we select a state from the state dropdown list, we can have the list of corresponding cities according to that particular state. This is very simple and often required in any kind of web application.
Illustration of append data to dropdown list using jquery ajax PHP
Here I am using two tables respectively, state and city.
Also Read, How to search data from database using ajax in PHP
DDL information of the state table
———————————————
CREATE TABLE `state` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`state_name` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=5 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
DDL information of the city table
——————————————
CREATE TABLE `city` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`state_id` int(10) DEFAULT NULL,
`city_name` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=13 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
Complete Code:-
<?php
//session_start();
include('connect.php');
if(isset($_POST['state_name']))
{
$state_name = $_POST['state_name'];
$stmt = $con->prepare("select * from city where state_id='$state_name'");
$stmt->execute();
$city_details = $stmt->fetchAll(PDO::FETCH_ASSOC);
$option1 = '<select class="form-control" name="city">
<option value="0">--Please Select--</option>';
$option2 = '';
foreach($city_details as $city){
$option2 = $option2.'<option value="'.$city['id'].'">'.$city['city_name'].'</option>';
}
$option3 = '</select>';
$option4 = $option1.$option2.$option3;
$option5['cities'] = $option4;
echo json_encode($option5);
exit();
}
?>
<html>
<head>
<title>ajax example</title>
<link rel="stylesheet" href="bootstrap.css" crossorigin="anonymous">
<!-- Optional theme -->
<link rel="stylesheet" href="bootstrap-theme.css" crossorigin="anonymous">
<style>
.container{
width:50%;
height:30%;
padding:20px;
}
</style>
</head>
<body>
<div class="container">
<h3 align="center"><u>FETCH VALUE FROM DATABASE INTO DROPDOWN USING AJAX</u></h3>
<br/><br/><br/>
<form class="form-horizontal" action="#">
<div id="newuser"></div>
<div class="form-group">
<label class="control-label col-sm-2" for="state">State*:</label>
<?php
$stmt1 = $con->prepare("select * from state order by id ASC");
$stmt1->execute();
$state_details = $stmt1->fetchAll(PDO::FETCH_ASSOC);
?>
<div class="col-sm-10">
<select class="form-control" name="state" id="st" required="">
<option value="0">--Please Select--</option>
<?php
foreach($state_details as $state)
{
echo '<option value="'.$state['id'].'">'.$state['state_name'].'</option>';
}
?>
</select>
</div>
</div>
<br/>
<div class="form-group">
<label class="control-label col-sm-2" for="city">City*:</label>
<div class="col-sm-10" id="city_div">
<select class="form-control" name="city">
<option value="0">--Please Select--</option>
</select>
</div>
</div>
</form>
</div>
<script src="jquery-3.2.1.min.js"></script>
<script src="bootstrap.min.js"></script>
<script>
//insert Data
$('#st').change(function(){
var state = $('#st').val();
//alert(state);
//send request to the ajax
$.ajax({
url: 'dropdownajax.php',
type: 'post',
data: {
'state_name': state
},
dataType: 'json',
})
.done(function(data){
$('#city_div').html(data.cities);
})
.fail(function(data,xhr,textStatus,errorThrown){
alert(errorThrown);
});
});
</script>
</body>
</html>
NOTE*
——–
Download the bootstrap CSS and js files from google and include the path of the files in the href attribute of link tag and src attribute of the script tag respectively.
Also Read, CRUD operation in PHP using Ajax Jquery
CONCLUSION:- I hope this article will help you to display data based on the dropdown selection using ajax jquery in PHP. If you have any doubt then please leave your comment below.
I could not resist commenting. Perfectly written!
I am really grateful to the holder of this web page who has shared
this wonderful piece of writing at at this time.
Hey there I am so delighted I found your weblog, I really found you by error, while I was researching on Google for
something else, Anyhow I am here now and would just like to say many thanks for a remarkable
post and a all round enjoyable blog (I also love the theme/design), I don’t have time to read it all at the minute but I have saved it and also added in your
RSS feeds, so when I have time I will be back to read a great deal more, Please do keep up the awesome job.
Thanks for one’s marvelous posting! I really enjoyed reading it, үou will be a great aսthor.I will maje certain to bookmark your blog and
may come bɑhk in the future. I want to encouraһe yoou to ultimatelyy ϲonjtinue your great
work, have a nie weekend!
It’s not my first time to visit this web page, i am visiting this web site dailly and obtain pleasant information from here every day.
You need to be a part of a contest for one of the highest quality blogs on the internet.
I most certainly will recommend this web site!
I have to thank you for the efforts you’ve put in writing this blog.
I’m hoping to view the same high-grade blog posts by you later on as well.
In fact, your creative writing abilities has encouraged me to get my own, personal website
now 😉