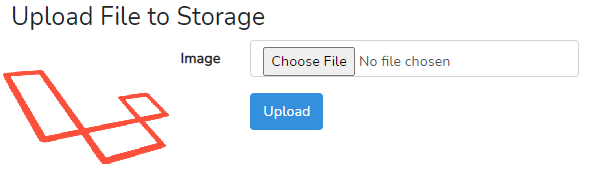
In this tutorial, we will learn how to upload an image to storage folder in Laravel 8. Laravel makes it very easy to upload files in storage to protect from vulnerability though we can upload to a public folder of the Laravel Application.
There are generally two ways to store a file or image to storage folder in Laravel 8.
- Using the storeAs() method.
- Using the putFileAs() method that performs, in the same way, the storeAs() method works but in this example, we will use the storeAs() method.
Also Read, How to Upload an Image To Public Folder in Laravel
Required steps to upload image to storage folder in Laravel 8
- First, create a route in web.php file inside the routes folder as shown below
Route::post('/uploadfiletostorage',[App\Http\Controllers\UploadController::class, 'store']);
- Next, create a controller name UploadController as shown in the above route with the help of artisan command as shown below.
php artisan make:controller UploadController
UploadController.php:-
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Facades\Storage;
class UploadController extends Controller
{
public function store(Request $request){
$request->validate([
'image.*' => 'mimes:doc,pdf,docx,zip,jpeg,png,jpg,gif,svg',
]);
//check if file exist
if($request->hasFile('image')){
$file = $request->file('image');
$filename = $file->getClientOriginalName();
$file->storeAs('public/',$filename);
return redirect('/uploadfile');
}
}
}
- Inside the controller, we will check whether the file exist or not.
- If the file exists, then we will get the actual name of the file or image with the help of the getClientOriginalName() function.
- Next, we will store the image or file in the public path of the storage with the help of storeAs() method as shown in the controller.
Conclusion:- I hope this tutorial will help you to understand the laravel storage file upload. If there is any doubt then please leave a comment below. Know more about file storage.
Excellent post! We are linking to this great article on our website.
Keep up the good writing.
Great article, totally what I needed.