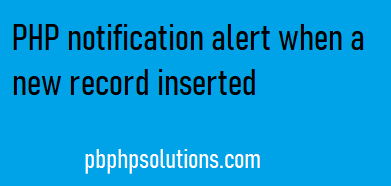
Hi friends, in this tutorial we will learn how to send a PHP notification alert when a new record is inserted using ajax and jquery. This is almost similar to Facebook-like notifications.
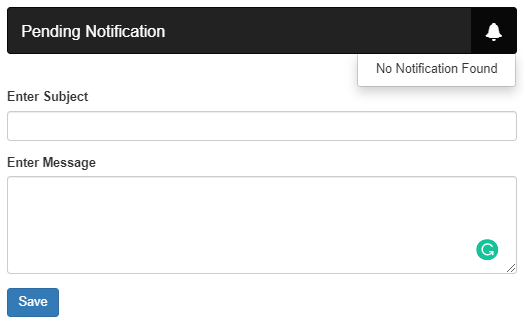
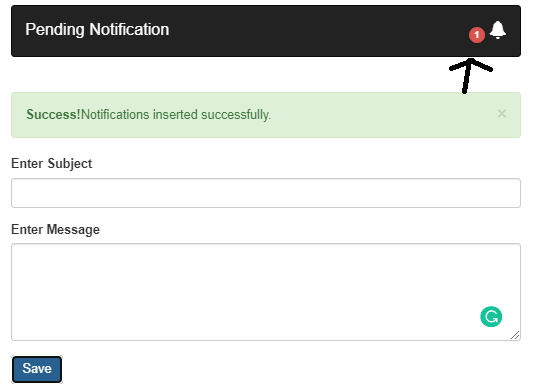
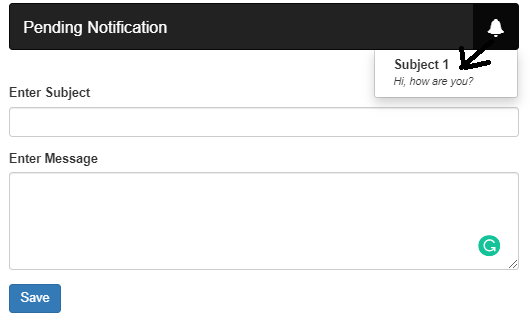
As we all know notification is a very essential part of any type of web-based application or any dynamic website. To do so, you have to follow the below-given steps one by one.
Also read, How to Get Only Date from Timestamp in PHP
Required steps of PHP notification alert
Step 1:- Create a table in the database.
DDL information of the table
CREATE TABLE `notifications` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`subject` varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`comment` varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`status` int(10) DEFAULT NULL,
`created_at` timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp(),
`updated_at` timestamp NOT NULL DEFAULT '0000-00-00 00:00:00',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=2 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
Step 2:- In this step, we will create a PHP file using an HTML form with two fields such as subject and comment, and save the file name as phpnotification.php.
phpnotification.php
<html>
<head>
<title>Notification System</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<style>
.container{
width:40%;
height:30%;
padding:20px;
margin-top: 5%;
}
</style>
</head>
<body>
<div class="container">
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" style="color: white;">Pending Notification</a>
</div>
<ul class="nav navbar-nav navbar-right">
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown"><span class="label label-pill label-danger count" style="border-radius:10px;"></span> <span class="glyphicon glyphicon-bell" style="font-size:20px;color: white;"></span></a>
<ul class="dropdown-menu"></ul>
</li>
</ul>
</div>
</nav>
<br />
<div id="show_notification"></div>
<form method="post" action="#">
<div id="error_div"></div>
<div class="form-group">
<label>Enter Subject</label>
<input type="text" name="subject" id="subject" class="form-control">
</div>
<div class="form-group">
<label>Enter Message</label>
<textarea name="comment" id="comment" class="form-control" rows="5"></textarea>
</div>
<div class="form-group">
<!--<input type="submit" name="post" id="post" class="btn btn-info" value="Post" />-->
<button type="button" class="btn btn-primary" name="post" id="post">Save</button>
</div>
</form>
</div>
</body>
</html>
Step 3:- In this step, we will send the form values with the help of the AJAX request i.e. without reloading the page using the done() function as shown below
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script>
$(document).ready(function(){
$('#post').click(function(){
//alert('ok');
var subject = $('#subject').val();
var comment = $('#comment').val();
if(subject=='' || comment==''){
$('#error_div').html('<div class="alert alert-danger"><strong>Please enter the required fields.</strong></div>');
}
else{
$.ajax({
url:"phpnotification.php",
method:"POST",
data:{'subject':subject,'comment':comment,'post':1},
dataType:"html"
})
.done(function(data){
load_unseen_notification();
$('#show_notification').html('<div class="alert alert-success alert-dismissible"><a href="#" class="close" data-dismiss="alert" aria-label="close">&times;</a><strong>Success!</strong>Notifications inserted successfully.</div>');
$('#subject').val('');
$('#comment').val('');
$('#error_div').html('');
})
.fail(function(xhr,textStatus,errorThrown,data)
{
alert(errorThrown);
});
}
});
});
</script>
Step 4:- In this step, we will receive the form values from the AJAX request and store them in the table of our database where the information will be stored.
Here, we will not create any separate PHP file for storing the data because we are using the same file for storing the form values with the help of PHP scripts as shown below.
Also, note that we are using a status field so that we can populate the unseen notifications after submitting the data depending on that status.
<?php
$servername='localhost';
$username="root";
$password="";
try
{
$con=new PDO("mysql:host=$servername;dbname=blog",$username,$password);
$con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
//echo 'connected';
}
catch(PDOException $e)
{
echo '<br>'.$e->getMessage();
}
if(isset($_POST["post"]))
{
$subject=$_POST['subject'];
$comment=$_POST['comment'];
$status = 0;
$sql="INSERT INTO notifications(subject,comment,status)VALUES('$subject','$comment','$status')";
$stmt=$con->prepare($sql);
$stmt->execute();
}
Step 5:- In this step, we are going to view the unseen notification in the dropdown menu after clicking the bell icon as shown in the HTML form.
Here, we have used the click() function with dropdown menu class and another function called load_unseen_notification() to call the notification information which is not seen yet as shown below.
$(document).on('click', '.dropdown-toggle', function(){
$('.count').html('');
load_unseen_notification('yes');
});
setInterval(function(){
load_unseen_notification();
}, 5000);
function load_unseen_notification(view = '')
{
$.ajax({
url:"fetchnotification.php",
method:"POST",
data:{view:view},
dataType:"json",
success:function(data)
{
$('.dropdown-menu').html(data.notification);
if(data.unseen_notification > 0)
{
$('.count').html(data.unseen_notification);
}
}
});
}
load_unseen_notification();
Step 6:- In this step, we will create a separate PHP file called fetchnotification.php which is used to collect the unseen notifications from the database and return the response back to the AJAX request sent from the function load_unseen_notification() as shown below.
fetchnotification.php:-
<?php
$servername='localhost';
$username="root";
$password="";
try
{
$con=new PDO("mysql:host=$servername;dbname=blog",$username,$password);
$con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
//echo 'connected';
}
catch(PDOException $e)
{
echo '<br>'.$e->getMessage();
}
if(isset($_POST['view'])){
if($_POST["view"] != '')
{
$stmt=$con->prepare("update notifications set status = 1 where status = 0");
$stmt->execute();
}
//Fetching the rows from database
$stmt1=$con->prepare("SELECT * FROM notifications ORDER BY id DESC LIMIT 5");
$stmt1->execute();
$output = '';
if($stmt1->rowCount() > 0)
{
while($row = $stmt1->fetch(PDO::FETCH_ASSOC))
{
$output .= '
<li>
<a href="#">
<strong>'.$row["subject"].'</strong><br />
<small><em>'.$row["comment"].'</em></small>
</a>
</li>
';
}
}
else{
$output .= '<li><a href="#" class="text-bold text-italic">No Notification Found</a></li>';
}
$stmt2=$con->prepare("SELECT * FROM notifications WHERE status = 0");
$stmt2->execute();
$count = $stmt2->rowCount();
$data = array(
'notification' => $output,
'unseen_notification' => $count
);
echo json_encode($data);
}
?>
Complete Code:-
<?php
$servername='localhost';
$username="root";
$password="";
try
{
$con=new PDO("mysql:host=$servername;dbname=blog",$username,$password);
$con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
//echo 'connected';
}
catch(PDOException $e)
{
echo '<br>'.$e->getMessage();
}
if(isset($_POST["post"]))
{
$subject=$_POST['subject'];
$comment=$_POST['comment'];
$status = 0;
$sql="INSERT INTO notifications(subject,comment,status)VALUES('$subject','$comment','$status')";
$stmt=$con->prepare($sql);
$stmt->execute();
}
?>
<html>
<head>
<title>Notification System</title>
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" />
<style>
.container{
width:40%;
height:30%;
padding:20px;
margin-top: 5%;
}
</style>
</head>
<body>
<div class="container">
<nav class="navbar navbar-inverse">
<div class="container-fluid">
<div class="navbar-header">
<a class="navbar-brand" style="color: white;">Pending Notification</a>
</div>
<ul class="nav navbar-nav navbar-right">
<li class="dropdown">
<a href="#" class="dropdown-toggle" data-toggle="dropdown"><span class="label label-pill label-danger count" style="border-radius:10px;"></span> <span class="glyphicon glyphicon-bell" style="font-size:20px;color: white;"></span></a>
<ul class="dropdown-menu"></ul>
</li>
</ul>
</div>
</nav>
<br />
<div id="show_notification"></div>
<form method="post" action="#">
<div id="error_div"></div>
<div class="form-group">
<label>Enter Subject</label>
<input type="text" name="subject" id="subject" class="form-control">
</div>
<div class="form-group">
<label>Enter Message</label>
<textarea name="comment" id="comment" class="form-control" rows="5"></textarea>
</div>
<div class="form-group">
<!--<input type="submit" name="post" id="post" class="btn btn-info" value="Post" />-->
<button type="button" class="btn btn-primary" name="post" id="post">Save</button>
</div>
</form>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.1.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
<script>
$(document).ready(function(){
$('#post').click(function(){
//alert('ok');
var subject = $('#subject').val();
var comment = $('#comment').val();
if(subject=='' || comment==''){
$('#error_div').html('<div class="alert alert-danger"><strong>Please enter the required fields.</strong></div>');
}
else{
$.ajax({
url:"phpnotification.php",
method:"POST",
data:{'subject':subject,'comment':comment,'post':1},
dataType:"html"
})
.done(function(data){
load_unseen_notification();
$('#show_notification').html('<div class="alert alert-success alert-dismissible"><a href="#" class="close" data-dismiss="alert" aria-label="close">&times;</a><strong>Success!</strong>Notifications inserted successfully.</div>');
$('#subject').val('');
$('#comment').val('');
$('#error_div').html('');
})
.fail(function(xhr,textStatus,errorThrown,data)
{
alert(errorThrown);
});
}
});
});
function load_unseen_notification(view = '')
{
$.ajax({
url:"fetchnotification.php",
method:"POST",
data:{view:view},
dataType:"json",
success:function(data)
{
$('.dropdown-menu').html(data.notification);
if(data.unseen_notification > 0)
{
$('.count').html(data.unseen_notification);
}
}
});
}
load_unseen_notification();
$(document).on('click', '.dropdown-toggle', function(){
$('.count').html('');
load_unseen_notification('yes');
});
setInterval(function(){
load_unseen_notification();
}, 5000);
</script>
</script>
</body>
</html>
Conclusion: I hope this tutorial will help you to understand the PHP notification system. If there is any doubt then please leave a comment below.