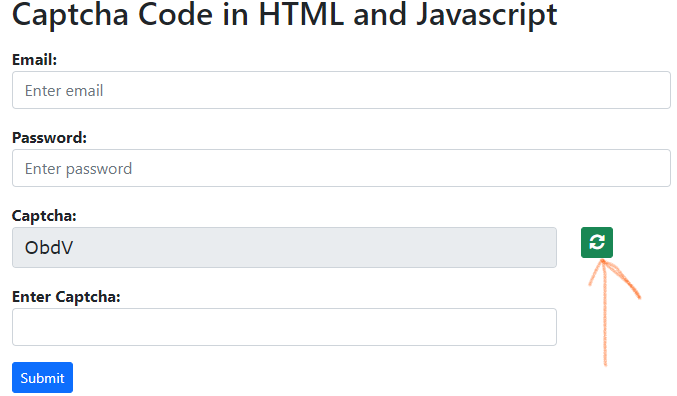
Hi friends, in this tutorial, you will learn how to create captcha code in HTML and Javascript. There are various ways to generate captchas such as Google ReCaptcha and other captchas etc.
Also read, Google Maps Add Marker Using Maps Javascript API and HTML
The logic of captcha code in HTML and Javascript
- First of all, we will call a function with the javascript onclick event handler to the input captcha field to generate the 4-digit random captcha code. The captcha code will load automatically when the page opens. Each time a captcha will be generated after clicking the refresh button.
- Now, we will use the javascript onsubmit event handler to the HTML form to validate the captcha. Suppose if a user hits the submit button without entering the captcha code then the function within the submit event handler will return false i.e. it prevents the HTML form to submit and an error message will be shown to the user.
- If you enter the wrong captcha then you will receive an error message otherwise you will receive a success alert for the correct captcha.
Also, you have to disable the copy text from the captcha field so that the captcha can not be copied. In order to do so, add the below lines of code inside the script tag.
// Disable right click on web page
$("html").on("contextmenu",function(e){
return false;
});
// Disable cut, copy and paste on web page
$('html').bind('cut copy paste', function (e) {
e.preventDefault();
});
Note:- Do not forget to include the jquery CDN link inside the head tag otherwise you can not disable the copy text feature.
HTML:-
<!DOCTYPE html>
<html lang="en">
<head>
<title>Captcha Code</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/css/bootstrap.min.css" rel="stylesheet">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.1.3/dist/js/bootstrap.bundle.min.js"></script>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/font-awesome/4.7.0/css/font-awesome.min.css">
</head>
<body onload="generateCaptcha(event);">
<div class="container mt-3" style="width:50%;">
<h2>Captcha Code in HTML and Javascript</h2>
<form action="/action_page.php" method="post" onsubmit="return validateForm()" name="myForm">
<div class="mb-3 mt-3">
<label for="email" class="fw-bold">Email:</label>
<input type="email" class="form-control" id="email" placeholder="Enter email" name="email">
</div>
<div class="mb-3">
<label for="pwd" class="fw-bold">Password:</label>
<input type="password" class="form-control" id="pwd" placeholder="Enter password" name="pswd">
</div>
<div class="mb-3">
<label for="pwd" class="fw-bold">Captcha:</label>
<div class="row">
<div class="col-sm-10">
<input type="text" name="maincaptcha" readonly class="form-control" id="mainCaptcha"/>
</div>
<div class="col-sm-2">
<button class="btn btn-success btn-sm" onclick="generateCaptcha(event);" id="refresh"><i class="fa fa-refresh" style="font-size:17px"></i></button>
</div>
</div>
</div>
<div class="mb-3">
<label for="pwd" class="fw-bold">Enter Captcha:</label>
<div class="row">
<div class="col-sm-10">
<input type="text" name="checkcaptcha" class="form-control" id="txtInput"/>
</div>
</div>
</div>
<div class="mb-3">
<span id="error" style="color:red;"></span>
<span id="success" style="color:green;"></span>
</div>
<button type="submit" class="btn btn-primary btn-sm">Submit</button>
</form>
</div>
<script type="text/javascript">
//for captcha
function generateCaptcha(event)
{
event.preventDefault();
var alpha = new Array('A','B','C','D','E','F','G','H','I','J','K','L','M','N','O','P','Q','R','S','T','U','V','W','X','Y','Z','a','b','c','d','e','f','g','h','i','j','k','l','m','n','o','p','q','r','s','t','u','v','w','x','y','z');
var i;
for (i=0;i<4;i++){
var a = alpha[Math.floor(Math.random() * alpha.length)];
var b = alpha[Math.floor(Math.random() * alpha.length)];
var c = alpha[Math.floor(Math.random() * alpha.length)];
var d = alpha[Math.floor(Math.random() * alpha.length)];
}
var code = a + '' + b + '' + '' + c + '' + d;
document.getElementById("mainCaptcha").value = code
var el = document.getElementById('mainCaptcha');
el.style.fontFamily = 'Geneva, Verdana, sans-serif';
el.style.fontSize = 'large';
}
//validate the form
function validateForm() {
let x = document.forms["myForm"]["checkcaptcha"].value;
let y = document.forms["myForm"]["maincaptcha"].value;
if (x == "") {
document.getElementById('error').innerHTML = "Please enter the captcha.";
return false;
}
if(x!==y){
document.getElementById('error').innerHTML = "Captcha does not match.";
return false;
}
if(x===y){
document.getElementById('error').innerHTML = "";
alert("Captcha entered successfully");
return true;
}
}
// automatically generate captcha after page laod
$(window).on('load', function () {
generateCaptcha(event);
});
// Disable right click on web page
$("html").on("contextmenu",function(e){
return false;
});
// Disable cut, copy and paste on web page
$('html').bind('cut copy paste', function (e) {
e.preventDefault();
});
</script>
</body>
</html>
Conclusion:- I hope this tutorial will help you to understand the concept of the captcha code. If there is any doubt then please leave a comment below.