Hi friends, in this tutorial, you will learn how to get value of a radio button using jQuery. This is a very simple process and we can do it with the help of javascript events like onclick event with a function inside the button HTML element and trigger that function within the script tag before the HTML body ends. In order to do this, you have to combine two types of attributes from the radio HTML element.
Steps to get value of a radio button using jQuery
Step 1:- Create an HTML file inside the root directory of your local server. In my case, it is a WAMP server.
Step 2:- Now, copy the below HTML code and paste it into the HTML file.
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title></title>
</head>
<body>
<h3>Get Value of a radio button using jQuery</h3>
<input type="radio" name="gender" value="Male">Male
<input type="radio" name="gender" value="Female">Female
<br><br>
<input type="button" value="select" onclick="getData()">
<br><br>
Gender = <input type="text" id="get_gender">
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
<script type="text/javascript">
function getData()
{
var gender = $("input[type='radio'][name='gender']:checked").val();
alert(gender);
$('#get_gender').val(gender);
}
</script>
</body>
</html>
Step 3:- Copy the jQuery CDN link from here.
Step 4:- Now, Copy the below javascript code and paste it into the HTML file before the end body tag.
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
<script type="text/javascript">
function getData()
{
var gender = $("input[type='radio'][name='gender']:checked").val();
alert(gender);
$('#get_gender').val(gender);
}
</script>
Complete Code:-
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title></title>
</head>
<body>
<h3>Get Value of a radio button using jQuery</h3>
<input type="radio" name="gender" value="Male">Male
<input type="radio" name="gender" value="Female">Female
<br><br>
<input type="button" value="select" onclick="getData()">
<br><br>
Gender = <input type="text" id="get_gender">
<script src="https://code.jquery.com/jquery-3.6.4.min.js"></script>
<script type="text/javascript">
function getData()
{
var gender = $("input[type='radio'][name='gender']:checked").val();
alert(gender);
$('#get_gender').val(gender);
}
</script>
</body>
</html>
Step 5:- Open your localhost in the browser and run the HTML file then you will see the form as shown on the below image.
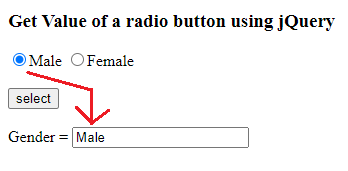
Conclusion:- I hope this will help you to understand the concept. If there is any doubt then please leave a comment below.