Hi friends, in this tutorial, you will learn how to select first 10 rows from MySQL using PHP. This kind of query is required very often when you need a certain number of rows from your MySQL database to display in the application or in the browser.
Also read, Delete a row in MySQL
There are various methods in MySQL such as PDO, MySQLi, etc by which we can do this. In order to do this, you can use two approaches of MySQL query as mentioned in the below steps.
Steps to select First 10 Rows from MySQL using PHP
Step 1:- First of all, create an HTML file inside the root directory of your local server.
Step 2:- Set up the database connection to your MySQL database as shown below
<?php
$servername='localhost';
$username="root";
$password="";
$dbname="myproject_db";
// Create connection
$con = mysqli_connect($servername, $username, $password, $dbname);
// Check connection
if (!$con) {
die("Connection failed: " . mysqli_connect_error());
}
?>
Step 3:- Create a table in the MySQL database as shown below
DDL information of the table
CREATE TABLE employee (
id int(10) NOT NULL AUTO_INCREMENT,
name varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL,
age int(20) NOT NULL,
department varchar(255) COLLATE utf8mb4_unicode_ci NOT NULL,
created_at timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
updated_at timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP,
PRIMARY KEY (id)
) ENGINE=MyISAM AUTO_INCREMENT=18 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
Step 4:- You must have some data in the table as shown below so that you can display those data with the help of different queries.
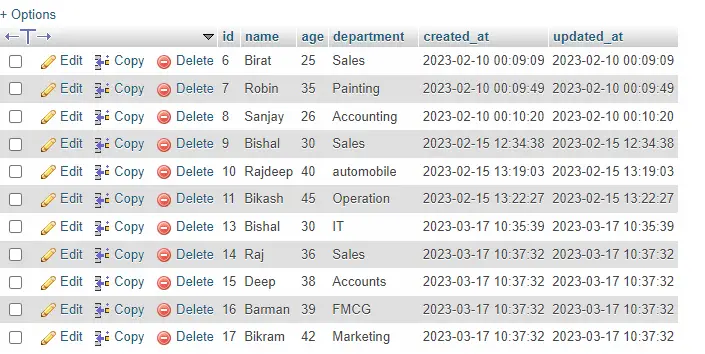
Step 5:- Now, get the first 10 rows from the table by using the below query with LIMIT as shown below
<?php
include 'dbconnect.php';
//get the first 10 rows from employee table using MySQLi
$sql3 = "SELECT * from employee ORDER BY id ASC limit 10";
$result = mysqli_query($con,$sql3);
?>
Step 6:- Also, you can get the first 10 rows from the table using the below query with comparison such as column name<= number value
<?php
include 'dbconnect.php';
//get the first 10 rows from employee table using MySQLi
$sql4 = "SELECT * from employee where id<=17 ORDER BY id ASC limit 10";
$result1 = mysqli_query($con,$sql4);
?>
From the above query, you have noticed that I have used id<=17 because my 11th row is 17
Step 7:- Now, you can display the data in an HTML table as shown in the below code
Complete Code:-
<?php
include 'dbconnect.php';
//get the first 10 rows from employee table using MySQLi
$sql3 = "SELECT * from employee ORDER BY id ASC limit 10";
$result = mysqli_query($con,$sql3);
$sql4 = "SELECT * from employee where id<=17 ORDER BY id ASC limit 10";
$result1 = mysqli_query($con,$sql4);
?>
<!DOCTYPE html>
<html>
<head>
<style>
table, th, td {
border: 1px solid black;
border-collapse: collapse;
}
</style>
</head>
<body>
<h2>Get first 10 rows from Employee Table</h2>
<table style="width:50%">
<tr>
<th>Sl No</th>
<th>Employee</th>
<th>Age</th>
<th>Department</th>
</tr>
<?php
if(mysqli_num_rows($result1)>0)
{
$inc = 1;
//output data for each row
while($row = mysqli_fetch_assoc($result))
{
echo '<tr>
<td>'.$inc++.'</td>
<td>'.$row['name'].'</td>
<td>'.$row['age'].'</td>
<td>'.$row['department'].'</td>
</tr>';
}
}
?>
</table>
</body>
</html>
Also read, Export Data From MySQL to Excel Using PHP
Conclusion:- I hope this tutorial will help you to understand the concept. If there is any doubt then please leave a comment below.