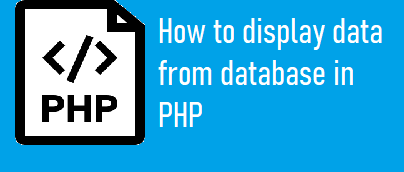
In this tutorial, you will learn how to display data from database in PHP. This is a very common feature and is required in any kind of web application. I will explain in detail from scratch. In order to get the table data from MySQL, we will use PHP code to retrieve data from MySQL database and display the result on our browser.
Also read, How to insert HTML form data in Mysql database using PHP
Steps to display data from database in PHP
Step 1:- Click on new at the left sidebar inside the phpMyAdmin.
Step 2:- Create a database in PHPMyAdmin as shown in the below screenshot.
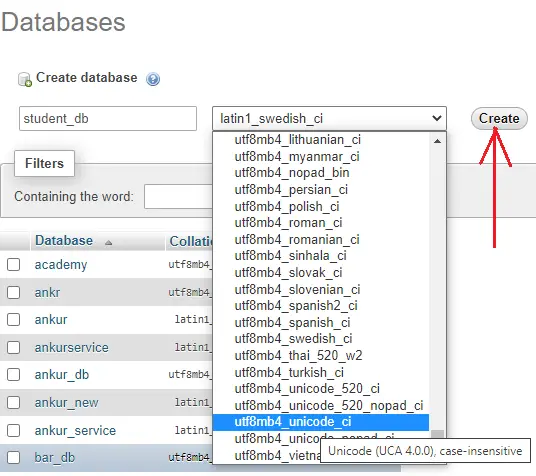
Step 3:- Create a table in the database you created as shown in the below screenshot.

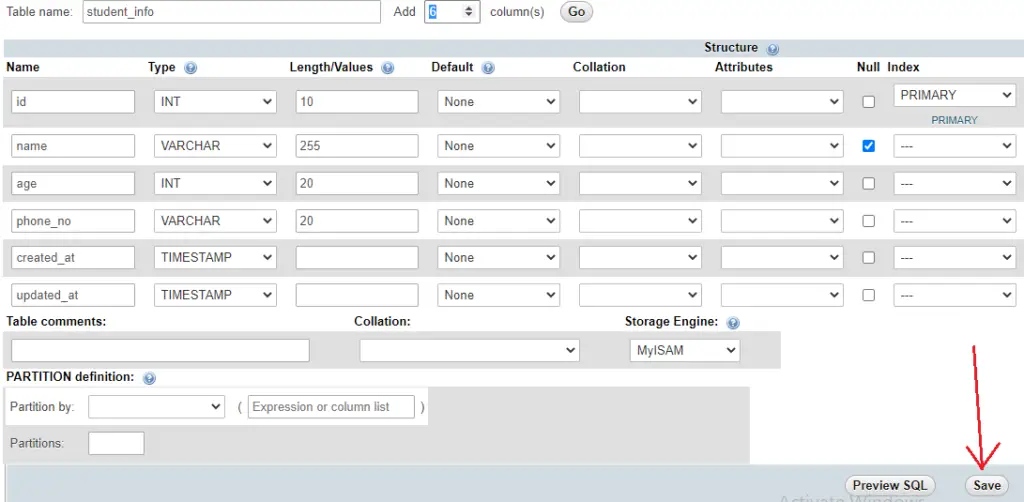
DDL information of the table
CREATE TABLE student_info (
id int(10) NOT NULL AUTO_INCREMENT,
name varchar(255) DEFAULT NULL,
age int(20) NOT NULL,
phone_no varchar(20) NOT NULL,
created_at timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp(),
updated_at timestamp NOT NULL DEFAULT '0000-00-00 00:00:00',
PRIMARY KEY (id)
) ENGINE=MyISAM AUTO_INCREMENT=4 DEFAULT CHARSET=latin1
Step 4:- Insert some data in the table manually as shown in the below screenshot.
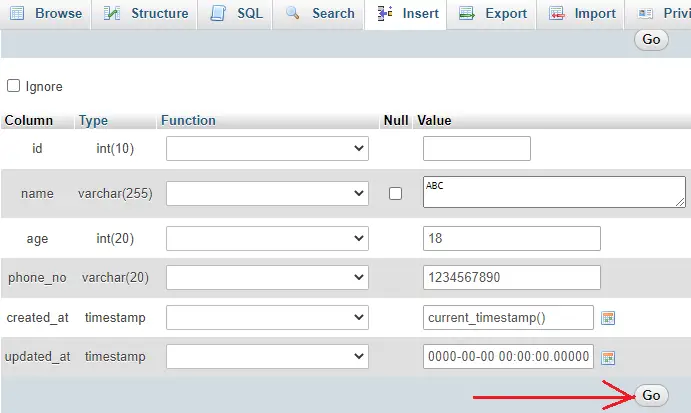
Step 5:- Also, you can insert data with the help of MySQL query as shown below
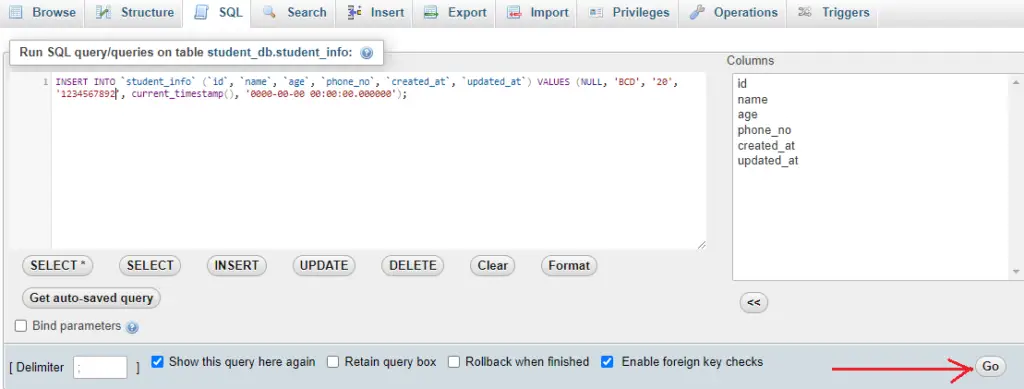
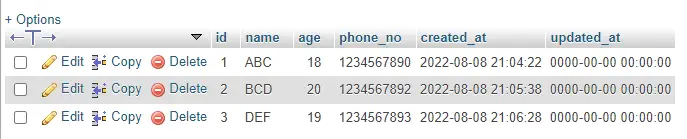
Step 7:- Now, make an HTML file inside the root directory of your local server with a .php extension so that we can insert the PHP script. HTML File:- (displaydata.php) mentioned in the last step.
Step 8:- Establish the database connection inside the HTML file with the help of PHP script as shown below
<?php
// Establish the connection with Mysql databae
$servername='localhost';
$username="root";
$password="";
try
{
$con=new PDO("mysql:host=$servername;dbname=student_db",$username,$password);
$con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
//echo 'connected';
}
catch(PDOException $e)
{
echo '<br>'.$e->getMessage();
}
?>
Step 9:- Now, write another PHP script to get the table data from the database as shown below
<?php
//PHP script to display the table data
$stmt = $con->prepare("select * from student_info");
$stmt->execute();
$student_details = $stmt->fetchAll(PDO::FETCH_ASSOC);
?>
Step 10:- Open the browser and hit the below URL
http://127.0.0.1:8000/displaydata
now, you will see the table data as shown below
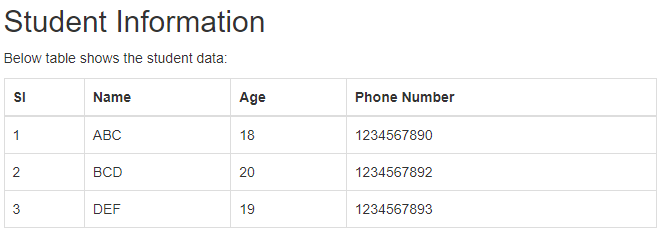
Complete Code:-
<?php
// Establish the connection with Mysql databae
$servername='localhost';
$username="root";
$password="";
try
{
$con=new PDO("mysql:host=$servername;dbname=student_db",$username,$password);
$con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
//echo 'connected';
}
catch(PDOException $e)
{
echo '<br>'.$e->getMessage();
}
//PHP script to display the table data
$stmt = $con->prepare("select * from student_info");
$stmt->execute();
$student_details = $stmt->fetchAll(PDO::FETCH_ASSOC);
?>
<!DOCTYPE html>
<html lang="en">
<head>
<title>Bootstrap Example</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
</head>
<body>
<div class="container" style="width:50%;">
<h2>Student Information</h2>
<p>Below table shows the student data:</p>
<table class="table table-bordered">
<thead>
<tr>
<th>Sl</th>
<th>Name</th>
<th>Age</th>
<th>Phone Number</th>
</tr>
</thead>
<tbody>
<?php
if($student_details)
{
foreach($student_details as $key=>$value)
{
?>
<tr>
<td><?php echo $key+1 ;?></td>
<td><?php echo $value['name'] ;?></td>
<td><?php echo $value['age'] ;?></td>
<td><?php echo $value['phone_no'] ;?></td>
</tr>
<?php
}
}
else{
?>
<tr>Data not found</tr>
<?php
}
?>
</tbody>
</table>
</div>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
</body>
</html>
Also, you can download the WAMP server to run programs locally.
Conclusion:- I hope this tutorial will help you to understand the overview. If there is any doubt then please leave a comment below