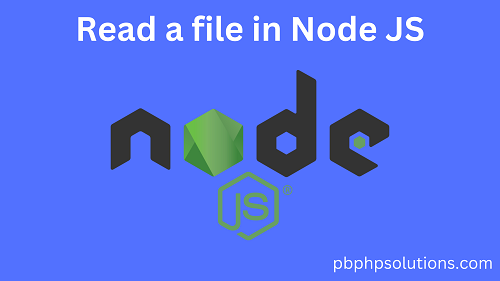
In this tutorial, you will learn how to read a file in Node JS. In order to do this, you have to include a built-in module of Node JS known as fs( File System) with the help of require() method as shown below
var fs = require("fs");
fs.readFile("name of the file","encoding","function(err,data))
Please note that the readFile() function contains three parameters
- the filename that has to be read using Node JS.
- Encoding defines the type of encoding format of the file that has to be read.
- The third parameter is a callback function that contains two arguments: err and data. If there is any error in your file then err will hold the error message. On the other hand, the data parameter will hold the contents of the file that has to be read if there is no error.
Also read, the Node JS HTTP server example
Steps to read a file in Node JS with an example
- Create an HTML file or a text file where the node JS file resides. (Mentioned Below)
- Put some contents in a file that has to be read.
- Now, create a Node JS file. (Mentioned Below)
- Open the command terminal and go to the location where your node JS file is stored and instantiate the node JS file as shown below
node filename.js
Now, press Enter.
You will see the output in your browser as shown below
Please read me with Node JS
This is a paragraph.
readfile.js
var http = require('http');
var fs = require('fs');
http.createServer(function(req,res){
//read the HTMl file
fs.readFile('test1.html','utf-8',function(err,data){
res.writeHead(200, {'Content-Type': 'text/html'});
res.write(data);
res.end();
});
}).listen(8080);
test1.html:-
<!DOCTYPE html>
<html>
<head>
<title>Page Title</title>
</head>
<body>
<h1>Please read me with Node JS</h1>
<p>This is a paragraph.</p>
</body>
</html>
Explanation of the above file
- First of all, I have included http and fs modules respectively. http is used to access data via hypertext transfer protocol which means over the web browser. On the other hand, the fs module is used to read the contents of the file.
- Now, the createServer() object is created because we have to get the response from the server.
- Now, pass the HTML file or any text file inside the readFile() function as the first parameter.
- Now, type the encoding format such as utf-8. This is optional.
- Now pass data inside the res.write(data) to display the result in the browser.
Also read, How to Import Custom Module in Node JS
Conclusion:- I hope this will help you to understand the concept. If there is any doubt then please leave a comment below.