Hello friends, in this tutorial you will learn how to render HTML data using Node JS. As I discussed earlier Node JS acts like a server in a javascript environment. It can handle multiple requests at once and return the responses to the client side without any delay.
Also read, Write a file into specific folder in Node JS example
In order to render HTML data, we will use express JS, a framework of Node JS that contains all the required packages and libraries to execute programs and handles the request data. If you do not know how to install express JS in vs code then click the below link.
Also Read, How to install Express js in vs code with an example
Required Steps to Render HTML Data using Node JS
Step 1:- Intialize all the express JS modules and packages as shown below.
const express = require('express');
In the above step, it is seen that I have used require(‘express’) to load the express modules and assigned it to a variable with the help of the const keyword.
Step 2:- Next, I have declared another variable data to use the functionality of express JS as shown below.
const data = express();
Step 3:- Now, we will pass the request and response parameter inside the get() method using the data variable as shown below.
data.get('/',(req,res)=>{
res.send('<h1>Welome to Home page</h1>');
});
In the above piece of code, you have seen that I have used a tag of HTML inside the send() function so that the Node JS server returns the content in an HTML form. Note that the send() is used with the response parameter.
data.get('/about',(req,res)=>{
res.send('<h3>Welome to about us page</h3>');
});
Similarly, I have used a tag in the above code.
data.get('/contact',(req,res)=>{
res.send('<h2>This is our contact us page.</h2><h4>You can contact me at 9876543210</h4>');
});
Similarly, I have used tag and tag in the above code to render the HTML data.
Complete Code:-
const express = require('express');
const data = express();
data.get('/',(req,res)=>{
res.send('<h1>Welome to Home page</h1>');
});
data.get('/about',(req,res)=>{
res.send('<h3>Welome to about us page</h3>');
});
data.get('/contact',(req,res)=>{
res.send('<h2>This is our contact us page.</h2><h4>You can contact me at 9876543210</h4>');
});
data.listen(3000);
Now, if you access the URL in your browser as localhost:3000 then you can see the below output.
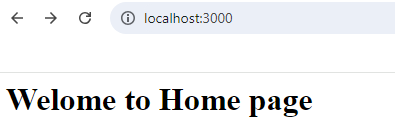
if you access the URL in your browser as localhost:3000/about then you can see the below output.
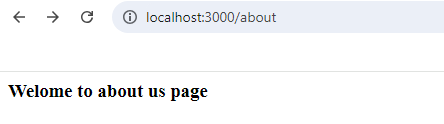
If you access the URL in your browser as localhost:3000/contact then you can see the below output.
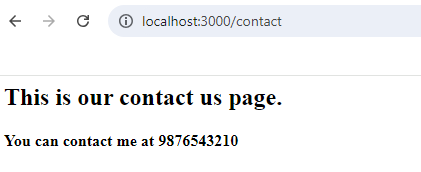
Conclusion:- I hope this tutorial will help you to understand the concept. If there is any doubt then please leave a comment below.