Hi friends, in this tutorial you will learn how to use the page loader jquery. This is very simple but often required while sending HTTP requests or any kind of request or submitting any form of data to let the user wait for the response till the response comes to the user.
Also, read, Get Value of a Radio Button Using jQuery
Steps to use page loader jQuery
Step 1:- Create an HTML file in the root directory of your local server i.e. www.
Step 2:- Paste the below code in the file.
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Page Loader!</title>
<style type="text/css">
#loader {
display: none;
position: fixed;
top: 0;
left: 50;
width: 100%;
height: 10%;
background-color: rgba(0, 0, 0, 0);
z-index: 9999;
}
</style>
</head>
<body>
<div class="container" style="width: 50%;">
<div id="loader"><div class="spinner-border text-info"></div> Please wait.........</div><br><br>
<h1>Page Loader jQuery Example !</h1>
<div class="mb-3">
<label for="exampleFormControlInput1" class="form-label">Name</label>
<input type="text" class="form-control" id="name">
</div>
<div class="mb-3">
<label for="exampleFormControlTextarea1" class="form-label">Phone Number</label>
<input type="number" class="form-control" id="phone_number">
</div>
<button type="button" class="btn btn-primary btn-sm frm_btn">Submit</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
</body>
</html>
Step 3:- Now, add the below javascript code or jquery code inside the script tag before the body tag ends in the above HTML file.
<script type="text/javascript">
$(document).ready(function(){
$('.frm_btn').click(function(){
// Show the loader
function showLoader() {
$('#loader').fadeIn('fast');
}
// Hide the loader
function hideLoader() {
$('#loader').fadeOut('fast');
}
showLoader();
setTimeout(function() {
hideLoader();
}, 3000);
});
});
</script>
Explanation of the above jquery code
- First of all, we will use the click() function or on-click event for the HTML file.
- When you click the submit button then it will call two functions respectively. One is showLoader() and another is hideLoader().
- Now, in the showLoader() function, I have called the fadeIn() method with the #loader div. This method makes a hidden element visible that means as soon as you click the submit button it will display the content or any kind of animation that exist in the loader div. Also, you have noticed that I have used a parameter “fast” inside the fadeIn() method to display the loader div content faster.
- Also, I have used another function called hideLoader(). So, inside this function, I have called another jquery method fadeOut(). This method makes a visible element to a hidden state that means as soon as you click the submit button, it will hide all the content of animation that exist in the loader div.
- Also, I have used a “fast” parameter to hide the loader div faster but in this case, I have used another method settimeout() for the hideLoader() function. As soon as the hideLoader() function is called the settimeout() method will be called automatically to hide the elements of the loader div based on the milliseconds declared inside the settimeout() method.
For 1 second = 1000 milliseconds.
Complete Code:-
<!doctype html>
<html lang="en">
<head>
<!-- Required meta tags -->
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<!-- Bootstrap CSS -->
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<title>Page Loader!</title>
<style type="text/css">
#loader {
display: none;
position: fixed;
top: 0;
left: 50;
width: 100%;
height: 10%;
background-color: rgba(0, 0, 0, 0);
z-index: 9999;
}
</style>
</head>
<body>
<div class="container" style="width: 50%;">
<div id="loader"><div class="spinner-border text-info"></div> Please wait.........</div><br><br>
<h1>Page Loader jQuery Example !</h1>
<div class="mb-3">
<label for="exampleFormControlInput1" class="form-label">Name</label>
<input type="text" class="form-control" id="name">
</div>
<div class="mb-3">
<label for="exampleFormControlTextarea1" class="form-label">Phone Number</label>
<input type="number" class="form-control" id="phone_number">
</div>
<button type="button" class="btn btn-primary btn-sm frm_btn">Submit</button>
</div>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.4/jquery.min.js"></script>
<script type="text/javascript">
$(document).ready(function(){
$('.frm_btn').click(function(){
// Show the loader
function showLoader() {
$('#loader').fadeIn('fast');
}
// Hide the loader
function hideLoader() {
$('#loader').fadeOut('fast');
}
showLoader();
setTimeout(function() {
hideLoader();
}, 3000);
});
});
</script>
</body>
</html>
If you access the file from your browser and click on the submit button then you can see the output as shown below.
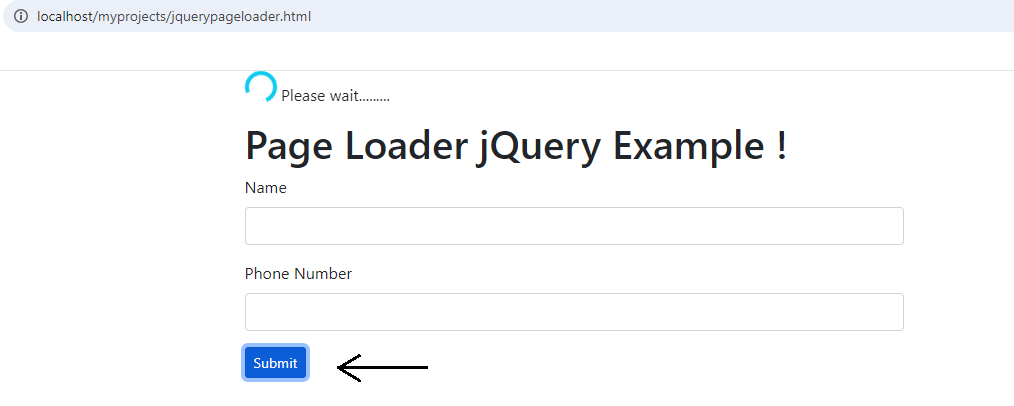
Conclusion:- I hope this tutorial will help you to understand the concept. If there is any doubt then please leave a comment below.