Hi friends, in this tutorial, you will learn how to render HTML file in express JS. As I told earlier that express JS is a framework of node JS with various packages and functionalities which reduces the code and execute the programs faster.
Also read, How to install Express js in vs code with an example
So, we will use the features of express JS by loading the modules of express JS in order to load HTML files. If you do not know how to run node JS programs then you can click the link below.
Also, read, Node Js hello world program
Required steps to render HTML file in express JS
Step 1:- Load the express JS module of node JS with the help of the const keyword as shown below.
const express = require('express');
Step 2:- Now, call the express() method to use the packages and functionalities of express JS and assigned it to a new variable called ‘data’ as shown below.
const data = express();
Step 3:- Next, we will initialize the path module of Node JS using the const keyword as shown below.
const path = require('path');
Please note that by using the path module we can get the path of any file or folder location.
Step 4:- Now, we will use the path module to get the path of the root directory of the project with the help of the join() method as shown below.
const publicPath = path.join(__dirname);
On the above line of code, join(__dirname) is used to get the path of the project directory.
Step 5:- Now, create a public folder under the root directory of the project as shown below.
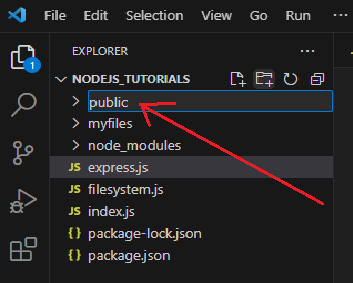
Step 6:- Now, we can access the path of the public folder inside the project directory by passing “public” as a second argument within the join() method as shown below.
const publicPath = path.join(__dirname,'public');
Step 7:- Create a simple HTML file inside the public folder as shown below.
about.html:-
<html>
<title>About US</title>
<head></head>
<body>
<h2>Welcome to about us page</h2>
</body>
</html>
Step 8:- Next, we will use the static() function of express JS modules and pass the publicPath as a parameter inside the static() function to access all the HTML files that exist in the public folder as given below.
data.use(express.static(publicPath));
Complete Code:- (express.js)
const express = require('express');
const data = express();
const path = require('path');
const publicPath = path.join(__dirname,'public');
console.log(publicPath);
data.use(express.static(publicPath));
data.listen(3000);
Step 9:- Go to your browser URL and type http://localhost:3000/about.html and press ENTER.
Step 10:- You will see the HTML file output as shown below.
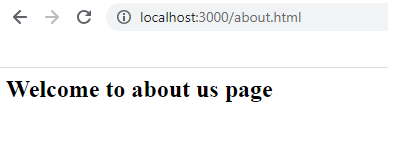
Conclusion:- I hope this tutorial will help you to understand the concept. If there is any doubt then please leave a comment below. Download vs code from here.