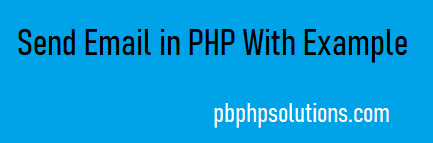
Hi friends, in this tutorial you will learn how to send email in PHP with the help of the mail() function. This function is used to send emails inside the PHP script. Email sending is a very common feature of any kind of web application and we must know it. Now, I am going to explain with an example using an HTML form as shown below
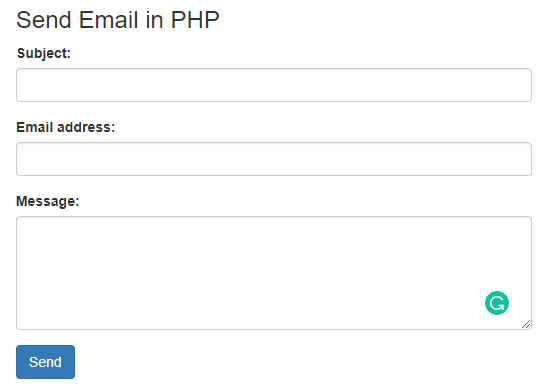
Send email in PHP using HTML form inputs
Before sending the emails, we must know the syntax of the mail() function as shown below
mail($to,$subject, $message, $headers)
whereas
$to = The email address of the receiver to whom the email will be sent.
$subject = The subject of the email (You can specify it directly in the PHP script or you can take the subject from the HTML input field)
$message = It defines the body text of the email ( You can specify directly in the PHP script or you can take the message text from the HTML input form)
$headers = In the headers section, there are multiple headers but you must set the From header. You can add an additional header like CC, BCC, etc. The headers should be separated with (r\n).
HTML Code:- (sendmail.html)
<!DOCTYPE html>
<html lang="en">
<head>
<title></title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
</head>
<body>
<div class="container" style="width: 40%;">
<br><br>
<h3>Send Email in PHP</h3>
<form method="post" action="send.php">
<div class="form-group">
<label for="email">Subject:</label>
<input type="text" name="subject" class="form-control form-control-sm">
</div>
<div class="form-group">
<label for="email">Email address:</label>
<input type="email" class="form-control form-control-sm" name="email">
</div>
<div class="form-group">
<label for="email">Message:</label>
<textarea name="message" class="form-control form-control-sm" rows="5"></textarea>
</div>
<div class="form-group">
<button type="submit" class="btn btn-primary" name="send">Send</button>
</div>
</form>
</div>
</body>
</html>
PHP Code:- (send.php)
<?php
if(isset($_POST['send']))
{
$subject = $_POST['subject'];
$email = $_POST['email'];
$message = $_POST['message'];
//set the email
$headers = "From: Sender Address senderemail\r\n";
$headers .= "Reply-To: senderemail\r\n";
$headers .= "Return-Path: senderemail\r\n";
//send the email
if(mail($to, $subject, $message, $headers)){
echo 'Email has been sent';
}
else{
echo 'Email could not send';
}
}
?>
Note:- mail() function will not work on your local server. It only works in a live server or shared hosting server.
Conclusion:- I hope this tutorial will help you to understand the concept of email sending. If there is any doubt then please leave a comment below.