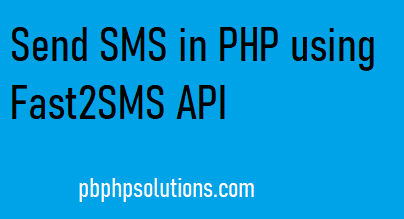
In this tutorial, I will explain how to send SMS in PHP using fast2sms API in a step-by-step process. SMS sending is a very important part of any kind of web application that provides instant information to users’ phone numbers to verify OTP and sending credentials etc. It is one of the most required features used by developers and can be integrated with the help of APIs provided by the SMS providers.
Fast2SMS is an SMS service provider that provides various SMS services such as bulk SMS, Quick SMS, DLT SMS, etc. Bulk SMS is used by companies, schools, and colleges, etc. In this tutorial, we will use the quick SMS service by using the quick SMS API in our application. In quick SMS, users will get SMS from random numeric numbers. After creating an account in Fast2SMS, Rs. 50 credited to the user account for free.
Also read, Integrate Instamojo payment gateway in PHP step by step
Prerequisite:- Any PHP version, XAMPP, or WAMP server.
Required steps to send SMS in PHP using FastSMS API
Step 1:- Create an account on the Fast2SMS website.
Step 2:- After creating the account, in the dashboard, navigate to the dev API at the left side of the dashboard and copy the API key for later use as shown below.
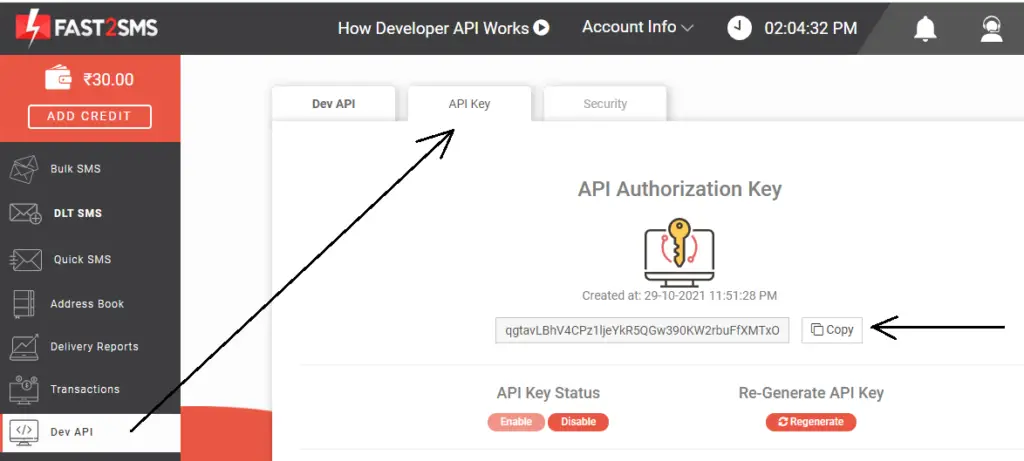
Step 3:- Just below the API key, go the API documentation and select the POST method from quick SMS API in PHP language as shown below.
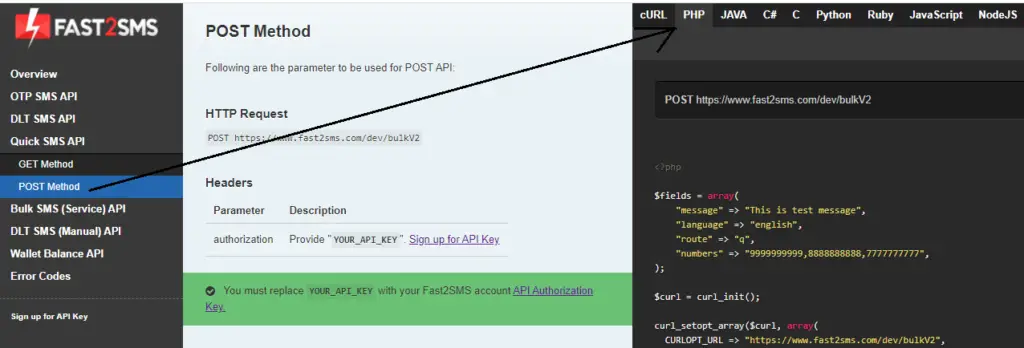
Step 4:- Now create a folder in the root directory of your local server. In my case, the folder name is smsinphp.
Step 5:- Now, create an index.php file inside the folder you have just created as shown below
index.php:-
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-EVSTQN3/azprG1Anm3QDgpJLIm9Nao0Yz1ztcQTwFspd3yD65VohhpuuCOmLASjC" crossorigin="anonymous">
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.bundle.min.js" integrity="sha384-MrcW6ZMFYlzcLA8Nl+NtUVF0sA7MsXsP1UyJoMp4YLEuNSfAP+JcXn/tWtIaxVXM" crossorigin="anonymous"></script>
<div class="container" style="width: 50%;">
<!-- Content here -->
<h5 class="text-center">Send SMS in PHP using fast2sms API</h5><hr><br>
<form method="post" action="send_sms.php">
<div class="mb-3">
<label for="exampleFormControlInput1" class="form-label fw-bold">Phone No*</label>
<input type="text" name="phone_no" class="form-control" id="exampleFormControlInput1">
</div>
<div class="mb-3">
<label for="exampleFormControlTextarea1" class="form-label fw-bold">Message</label>
<textarea class="form-control" name="message" id="exampleFormControlTextarea1" rows="3"></textarea>
</div>
<div class="mb-3">
<button type="submit" name="submit" class="btn btn-primary">Submit</button>
</div>
</form>
</div>
<script src="https://cdn.jsdelivr.net/npm/@popperjs/core@2.9.2/dist/umd/popper.min.js" integrity="sha384-IQsoLXl5PILFhosVNubq5LC7Qb9DXgDA9i+tQ8Zj3iwWAwPtgFTxbJ8NT4GN1R8p" crossorigin="anonymous"></script>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.0.2/dist/js/bootstrap.min.js" integrity="sha384-cVKIPhGWiC2Al4u+LWgxfKTRIcfu0JTxR+EQDz/bgldoEyl4H0zUF0QKbrJ0EcQF" crossorigin="anonymous"></script>
Step 6:- Now create a PHP action file send_sms.php separately that was mentioned in the index.php file as shown below.
send_sms.php:-
<?php
if(isset($_POST['submit'])){
//get the inputs
$phone = $_POST['phone_no'];
$message = $_POST['message'];
$fields = array(
"message" => $message,
"language" => "english",
"route" => "q",
"numbers" => $phone,
);
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => "https://www.fast2sms.com/dev/bulkV2",
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => "",
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 30,
CURLOPT_SSL_VERIFYHOST => 0,
CURLOPT_SSL_VERIFYPEER => 0,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => "POST",
CURLOPT_POSTFIELDS => json_encode($fields),
CURLOPT_HTTPHEADER => array(
"authorization: your api key from fast2sms",
"accept: */*",
"cache-control: no-cache",
"content-type: application/json"
),
));
$response = curl_exec($curl);
$err = curl_error($curl);
curl_close($curl);
if ($err) {
echo "cURL Error #:" . $err;
} else {
/*echo '<pre>';
echo $response;*/
echo '<b>SMS sent successfully on the number: '.$phone.'</b>';
header("refresh:5;url=index.php");
}
}
?>
Illustration of the above code:-
- First of all, we will receive the form inputs with the help of PHP global variable $_POST and store.
- Next, we will put the input fields in an array. The array index “route”=>”q” is used for sending quick SMS through quick SMS API that is available 24*7.
- Now, we will put our API key as mentioned earlier in the CURLOPT_HTTPHEADER array as “authorization”.
- Now, if you echo the response in the else condition of the last part of the above code then you can see the response as shown below
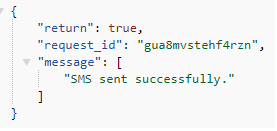
Use the above code snippet just for checking the response of the message.
Step 6:- Now open the browser and enter the URL as shown below.
http://localhost/myprojects/smsinphp/index.php
Now, you can send the SMS as shown below
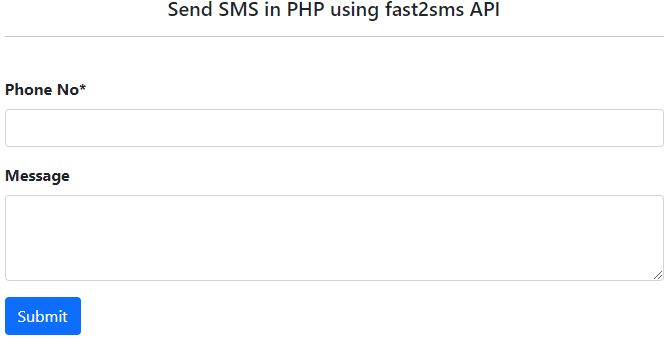
Conclusion:- I hope this tutorial will help you to understand the concept of SMS sending. If there is any doubt then please leave a comment below.