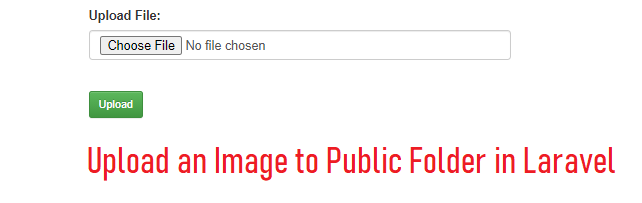
In this tutorial, we will learn how to upload an image to public folder in Laravel. Usually, there are two ways to upload a file in Laravel.
- Upload into the public folder of Laravel is very easy.
- Upload files into Laravel Storage that is also very easy.
Also read, How to create laravel project from scratch step by step
Required Steps to upload an image in Laravel
- Create routes in the web.php file inside the routes folder of the Laravel Application as given below
Route::get('/uploadfile','UploadController@index');
Route::post('/uploadfile','UploadController@store');
- Create a controller using the artisan command in the command terminal as shown below
php artisan make:controller UploadController
UploadController.php:-
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use DB;
use Auth;
use File;
class UploadController extends Controller
{
/**
* Display a listing of the resource.
*
* @return \Illuminate\Http\Response
*/
public function index()
{
return view('uploadfile');
}
public function store(Request $request)
{
//
$request->validate([
'image.*' => 'mimes:doc,pdf,docx,zip,jpeg,png,jpg,gif,svg',
]);
if($file = $request->hasFile('image')) {
$file = $request->file('image') ;
$fileName = $file->getClientOriginalName() ;
$destinationPath = public_path().'/images' ;
$file->move($destinationPath,$fileName);
return redirect('/uploadfile');
}
}
}
- Create a blade file to upload the file inside the resources/views folder of the Laravel Application to upload the file. I have created a blade file name ‘uploadfile.blade.php’.
- Do not forget to use the enctype=”multipart/form-data” in the form of the blade file.
uploadfile.blade.php:-
@include('layout.partials.head')
@include('layout.partials.sidemenu')
<h5>Upload the File</h5>
<hr>
<form action="/uploadfile" method="post" enctype="multipart/form-data">
{{ csrf_field() }}
<div class="form-group row">
<label for="Image" class="col-sm-2 col form-label" style="font-weight: bold;">Serial No</label>
<div class="col-sm-4">
<input type="file" class="form-control form-control-sm" name="image">
</div>
</div>
@if ($errors->any())
<div class="alert alert-danger">
<ul>
@foreach ($errors->all() as $error)
<li>{{ $error }}</li>
@endforeach
</ul>
</div>
@endif
<button type="submit" class="btn btn-primary btn-sm">Submit</button>
</form>
@include('layout.partials.footer')
Illustration of the example to upload an image to public folder in Laravel
- First of all, we have to create a custom folder inside the public folder in Laravel application.
- Next, we will validate the file request to know whether it is a file type or not as shown below
$request->validate([
'product_image.*' => 'mimes:doc,pdf,docx,zip,jpeg,png,jpg,gif,svg',
]);
- If the request has the file type then we will get the file name using getClientOriginalName() function.
- Then we will move the file to the folder we have created inside the public folder of Laravel.
Conclusion:- I hope this tutorial will help you to upload file in laravel. If you have any doubt then please leave a comment below. Know more about file uploading in Laravel
Fantastic items from you, man. I have understand your stuff previous to
and you are simply too great. I really like what
you’ve received here, really like what you’re saying and
the way in which you assert it. You’re making it entertaining and you still care for to stay it wise.
I can’t wait to read much more from you. That is really a terrific website.
My spouse and I stumbled over here by a different web address and thought I may as well check
things out. I like what I see so i am just following you.
Look forward to checking out your web page repeatedly.
Hi I am so glad I found your blog page, I really found
you by error, while I was researching on Aol for something else,
Anyhow I am here now and would just like to say kudos for a tremendous post and a all round interesting blog (I
also love the theme/design), I don’t have time to browse it all at the moment but I have saved it
and also included your RSS feeds, so when I have time I will be
back to read a lot more, Please do keep up the fantastic job.
Hey there, You have done an excellent job.
I will certainly digg it and personally suggest to my friends.
I’m sure they’ll be benefited from this website.