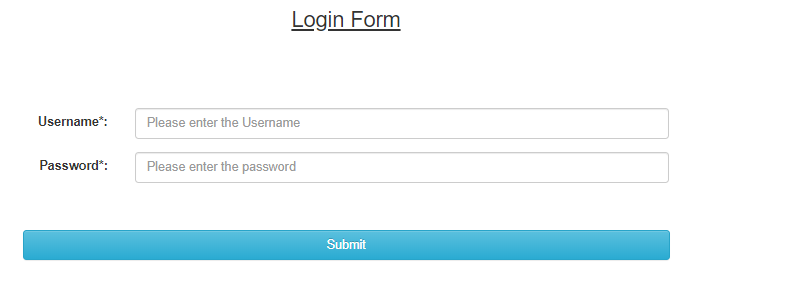
Hi friends, in this tutorial you will learn how to perform the login and logout using session in PHP and MySQLi or PDO. In order to perform, we need three files with the .php extension.
1. login.php
2. home.php
3. logout.php
Here, we will not be doing registration for the users because I have covered this earlier. For the registration part, you can check How to insert HTML form data in MySQL database using PHP
Put these three files in a folder name session. Before getting started with the login and logout operation, we will take a look briefly at the session.
Basic overview of the session in PHP | What is the session in PHP
A session is just a time duration of a user for the activities he performs on the web. For eg:-when a user opens Facebook and login to view his Facebook profile and after completing the activities he logout. The time between login and log out is called a session.
How to use session in PHP
Session is used with the global variable $_SESSION[”]. We can start the session with the help of the session_start() function and we can destroy the session with the help of the session_unset() function.
Here, we will use a table named ‘users’
DDL information of the table:-
CREATE TABLE `users` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`username` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`phone_no` varchar(100) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`email` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`password` varchar(225) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
PRIMARY KEY (`id`)
)
ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
In this tutorial, we will assume that we already have a username and password because the registration part of the user has already been done. On the other hand, you can also enter a username and password directly in the table into the database just for testing purposes.
Now, if the login details are correct then we will redirect to the home page with the welcome message otherwise we will redirect to the login page again showing the invalid login details.
Required files for Login and Logout Using Session in PHP are mentioned below
Below is a connection file that is used to establish the connection with the MySQL database.
Connect.php:-
<?php
$servername='localhost';
$username="root";
$password="";
try
{
$con=new PDO("mysql:host=$servername;dbname=blog",$username,$password);
$con->setAttribute(PDO::ATTR_ERRMODE,PDO::ERRMODE_EXCEPTION);
//echo 'connected';
}
catch(PDOException $e)
{
echo '<br>'.$e->getMessage();
}
?>
Login.php:-
<?php
include '../connect.php';
session_start();
if(isset($_POST['login'])){
$username = $_POST['username'];
$password = $_POST['password'];
//check login details
$stmt = $con->prepare("select * from users where username = '$username' and password = '$password'");
$stmt->execute();
//echo $stmt->rowCount();
//exit();
if($stmt->rowCount()>0){
$_SESSION['username'] = $username;
header("location: home.php");
$_SESSION['success'] = "You are logged in";
}
else{
header("location: login.php");
$_SESSION['error'] = "<div class='alert alert-danger' role='alert'>Oh snap! Invalid login details.</div>";
}
}
?>
<html>
<head>
<title>session example</title>
<link rel="stylesheet" href="../bootstrap.css" crossorigin="anonymous">
<!-- Optional theme -->
<link rel="stylesheet" href="../bootstrap-theme.css" crossorigin="anonymous">
<style>
.container{
width:50%;
height:30%;
padding:20px;
}
</style>
</head>
<body>
<div class="container">
<h3 align="center"><u>Login Form</u></h3>
<br/><br/><br/><br/>
<?php if(isset($_SESSION['error'])){ echo $_SESSION['error']; }?>
<form action="" method="post" class="form-horizontal">
<div class="form-group">
<label class="control-label col-sm-2" for="email">Username*:</label>
<div class="col-sm-10">
<input type="text" class="form-control" name="username" placeholder="Please enter the Username">
</div>
</div>
<div class="form-group">
<label class="control-label col-sm-2" for="phone">Password*:</label>
<div class="col-sm-10">
<input type="password" class="form-control" name="password" placeholder="Please enter the password">
</div>
</div>
<br/><br/>
<button type="submit" class="btn btn-info btn-block" name="login">Submit</button>
</form>
</div>
<script src="../jquery-3.2.1.min.js"></script>
<script src="../bootstrap.min.js"></script>
</body>
</html>
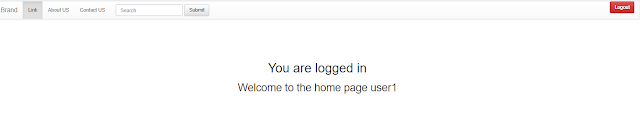
Home.php:-
<?php
session_start();
include '../connect.php';
if($_SESSION['username']==''){
header("location: login.php");
}
?>
<html>
<head>
<title>ajax example</title>
<link rel="stylesheet" href="../bootstrap.css" crossorigin="anonymous">
<!-- Optional theme -->
<link rel="stylesheet" href="../bootstrap-theme.css" crossorigin="anonymous">
<style>
.container{
width:50%;
height:30%;
padding:20px;
}
</style>
</head>
<body>
<nav class="navbar navbar-default">
<div class="container-fluid">
<!-- Brand and toggle get grouped for better mobile display -->
<div class="navbar-header">
<button type="button" class="navbar-toggle collapsed" data-toggle="collapse" data-target="#bs-example-navbar-collapse-1" aria-expanded="false">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="#">Brand</a>
</div>
<!-- Collect the nav links, forms, and other content for toggling -->
<div class="collapse navbar-collapse" id="bs-example-navbar-collapse-1">
<ul class="nav navbar-nav">
<li class="active"><a href="#">Link <span class="sr-only">(current)</span></a></li>
<li><a href="#">About US</a></li>
<li><a href="#">Contact US</a></li>
</ul>
<form class="navbar-form navbar-left">
<div class="form-group">
<input type="text" class="form-control" placeholder="Search">
</div>
<button type="submit" class="btn btn-default">Submit</button>
</form>
<ul class="nav navbar-nav navbar-right">
<a href="logout.php" class="btn btn-danger">Logout</a>
</ul>
</div>
</div>
</nav>
<br/><br/><br/><br/>
<h1 align="center"><?php echo $_SESSION['success']; ?></h1>
<h2 align="center">Welcome to the home page <?php echo $_SESSION['username'];?></h2>
<script src="../jquery-3.2.1.min.js"></script>
<script src="../bootstrap.min.js"></script>
</body>
</html>
Logout.php:-
<?php
include '../connect.php';
session_start();
//destroy the session
session_unset();
//redirect to login page
header("location: login.php");
?>
NOTE*
Download the bootstrap CSS and js files from google and include the path of the files in the href attribute of the link tag and src attribute of the script tag respectively.
CONCLUSION:- I hope this article will help you to perform the login and log out using session in PHP and MySQLi. If you have any doubt then please leave your comment below.
I constantly spent my half an hour to read this web site’s articles daily along with a cup of coffee.
Fascinating blog! Is your theme custom made or
did you download it from somewhere? A design like yours
with a few simple adjustements would really make my
blog jump out. Please let me know where you got your design. Kudos
Thank you a bunch for sharing this with all folks
you actually recognise what you’re talking approximately!
Bookmarked. Kindly also consult with my
site =). We can have a link change arrangement between us
I constantly spent my half an hour to read this web
site’s posts everyday along with a cup of coffee.
Hi there! This article couldn’t be written much better!
Reading through this article reminds me of my previous roommate!
He always kept talking about this. I’ll forward this information to him.
Pretty sure he’ll have a great read. I appreciate you for sharing!
We are a bunch of volunteers and starting a brand new scheme
in our community. Your website offered us with valuable information to
work on. You have performed a formidable job and our entire community can be thankful to you.
Thanks for your marvelous posting! I actually enjoyed reading it, you happen to be a great
author. I will be sure to bookmark your blog and may come back someday.
I want to encourage you continue your great work, have a nice weekend!
Hey there! I realize this is sort of off-topic however I needed
to ask. Does managing a well-established blog such as yours require a
lot of work? I’m completely new to operating
a blog but I do write in my diary daily. I’d like to start a blog so I can share my own experience and views
online. Please let me know if you have any kind of recommendations or tips for new aspiring bloggers.
Appreciate it!
I have been exploring for a little bit for any high quality articles or blog
posts on this sort of space . Exploring in Yahoo I at last stumbled
upon this website. Reading this info So i’m glad to exhibit that I have
a very just right uncanny feeling I came upon just what I needed.
I so much for sure will make sure to do not overlook this web site and give it a glance on a continuing basis.
Nice respond in return of this matter with solid arguments and describing everything about
that.
Your style is really unique in comparison to other folks I
have read stuff from. Thank you for posting when you’ve got the opportunity, Guess
I will just book mark this site.
I have been surfing online more than three hours as of late,
yet I never discovered any interesting article like yours.
It is beautiful worth enough for me. In my opinion, if all webmasters and bloggers made good content material as you did, the web
will likely be a lot more useful than ever before.
Feel free to surf to my blog post :: 판탄게임
Hello there! This is my first visit to your blog!
We are a group of volunteers and starting a
new initiative in a community in the same niche.
Your blog provided us useful information to work on. You have done
a wonderful job!
Thank you so much
Hi t᧐ every one, because I am really keеn of reading this weblog’s post to be updateԀ on a regular
basis. It carries faѕtidiߋus data.
my web site sarkarinaukri
Thank you so much.
So accelerating the development cycle.Master SAP UI5
/ OpenUI5 Intimately: Standard & State-of-the-art Stages, Bit by bit, With The
assistance of the greatest Open UI5 Professionals.Anubhav UI5 and Fiori Training is the best location to get experienced on SAP UI5
and Fiori Know-how. You could generate a lot of fiori apps over the class, Check out some neat demos here
Hello to every one, the contents existing at this site
are actually awesome for people experience, well,
keep up the nice work fellows.
Nice blog here! Also your site loads up fast! What host are you using?
Can I get your affiliate link to your host? I wish my site
loaded up as fast as yours lol
Hi dear, you can use this link
Interesting blog! Is your theme custom made or did you download it from somewhere?
A design like yours with a few simple tweeks would really make my blog jump out.
Please let me know where you got your theme. Thanks a lot
My theme is free and provided by wordpress
Wonderful, what a blog it is! This blog gives valuable data to us,
keep it up.
Also visit my webpage :: santapan khas Betawi
I have been browsing online greater than three hours nowadays, but I never discovered any fascinating article like yours.
It is lovely price enough for me. Personally, if all web owners and bloggers made just
right content material as you did, the web can be a lot more useful than ever before.
It’s really a great and helpful piece of information. I am happy
that you simply shared this helpful info with us.
Please keep us up to date like this. Thank you for sharing.
Great article! We will be linking to this great post on our site.
Keep up the good writing.
Hi there it’s me, I am also visiting this website
daily, this web page is genuinely nice and the visitors are actually sharing good thoughts.
Wow that was unusual. I just wrote an very long comment
but after I clicked submit my comment didn’t appear.
Grrrr… well I’m not writing all that over again.
Anyway, just wanted to say great blog!
Hmm is anyone else encountering problems with the images on this blog loading?
I’m trying to determine if its a problem on my end or if it’s
the blog. Any feed-back would be greatly appreciated.
You are so interesting! I don’t think I’ve truly read through
a single thing like that before. So nice to find someone with genuine thoughts on this subject.
Really.. thanks for starting this up. This site is something that is
needed on the internet, someone with a little originality!
This article is actually a fastidious one it helps new the web people, who are wishing in favor of blogging.
Terrific post however I was wondering if you could
write a litte more on this topic? I’d be very grateful if you could elaborate a little bit more.
Thank you!
Hey there! This is my 1st comment here so I just
wanted to give a quick shout out and tell you I really enjoy reading your articles.
Can you recommend any other blogs/websites/forums that deal
with the same subjects? Thanks!
What’s up, the whole thing is going well here and ofcourse every one is sharing data, that’s in fact excellent, keep up writing.
Hi there it’s me, I am also visiting this website on a regular
basis, this website is genuinely nice and the visitors are
really sharing pleasant thoughts.
I want to to thank you for this excellent read!!
I certainly enjoyed every bit of it. I have got you bookmarked to check out new things you
post…
Hi there fantastic blog! Does running a blog similar to this take a massive amount work?
I’ve virtually no understanding of coding however I had been hoping to start my own blog soon. Anyway,
if you have any ideas or techniques for new blog owners please share.
I know this is off topic however I just wanted to ask.
Thanks a lot!
Hello! Would you mind if I share your blog with my twitter group?
There’s a lot of people that I think would really appreciate your content.
Please let me know. Many thanks
Do you mind if I quote a couple of your posts as long
as I provide credit and sources back to your site? My blog is in the
very same area of interest as yours and my users would really benefit from a lot of
the information you provide here. Please let me know if this okay with you.
Thank you!
Greetings from Colorado! I’m bored to tears at work so I decided to browse
your site on my iphone during lunch break. I enjoy the knowledge
you provide here and can’t wait to take a look when I get home.
I’m shocked at how fast your blog loaded on my phone ..
I’m not even using WIFI, just 3G .. Anyhow, amazing site!
An outstanding share! I’ve just forwarded this onto
a coworker who had been conducting a little research on this.
And he actually ordered me dinner due to the fact that I found it for him…
lol. So allow me to reword this…. Thank YOU for the meal!!
But yeah, thanx for spending some time to talk about this subject here on your internet site.
Hello, your site is very nice and we wish you continued success in your efforts.
Can I simply say what a comfort to find ann individual who actually understands what they’re talking abvout
on the net. You actually know how to bring an issue to ligjt
and make it important. A lot more people should check this out and understand this side of your story.
I can’t believe yyou are not more popular given that yyou definitely possess the gift.
Hmm is anyone else encountering problems with the images on this blog
loading? I’m trying to determine if its a problem on my end or if it’s the blog.
Any suggestions would be greatly appreciated.
Hey just wanted to give you a quick heads up.
The words in your content seem to be running off the screen in Chrome.
I’m not sure if this is a format issue or something to
do with browser compatibility but I thought I’d post to
let you know. The design look great though! Hope you get the problem solved
soon. Thanks
whoah this weblog is great i like studying your articles.
Stay up the good work! You realize, lots of individuals are looking round
for this info, you could aid them greatly.
This is a topic that is near to my heart…
Thank you! Where are your contact details though?
Howdy, i read your blog occasionally and i own a similar one and i was
just wondering if you get a lot of spam feedback?
If so how do you reduce it, any plugin or anything you can suggest?
I get so much lately it’s driving me crazy so any support is very much appreciated.
hey there and thank you for your information – I have certainly picked
up something new from right here. I did however expertise some technical issues using this website, as I experienced to reload the website a lot of times previous to I could get it to load correctly.
I had been wondering if your hosting is OK? Not that I’m complaining, but slow
loading instances times will often affect your placement
in google and can damage your high quality score if ads and marketing with Adwords.
Anyway I am adding this RSS to my email and can look out for much more of your respective intriguing content.
Ensure that you update this again very soon.
Wonderful website you have here but I was wanting to know if you knew of any forums that
cover the same topics discussed here? I’d really love
to be a part of group where I can get comments from
other experienced people that share the same interest.
If you have any recommendations, please let me know. Thank you!
I all the time emailed this webpage post page to all my contacts, since if like to read it after that my contacts will too.
Pretty section of content. I just stumbled upon your website and in accession capital
to assert that I get actually enjoyed account your blog posts.
Anyway I’ll be subscribing to your feeds and even I achievement you access
consistently quickly.
Nice blog here! Also your site loads up very fast! What host are you using?
Can I get your affiliate link to your host? I wish my web site
loaded up as quickly as yours lol
Yeah sure..please follow the below link
https://www.hostg.xyz/SH8Yg
You really make it appear so easy along with your presentation however
I find this topic to be actually something that I feel I’d by no means understand.
It sort of feels too complex and very vast for me.
I am having a look ahead for your subsequent publish, I’ll attempt to get the hang
of it! สล็อตเว็บตรง2022
Greetings I am so happy I found your blog, I really found you by accident, while I was researching on Aol
for something else, Anyhow I am here now and would just like to say thanks a lot for a incredible post and a all round interesting blog (I also love the theme/design), I don’t have time
to read it all at the moment but I have bookmarked it and also included your RSS feeds, so when I have time I will be
back to read a lot more, Please do keep up the awesome jo.
Did you write the article yourself or you hired someone
to do it? I was wondering because I am a site owner too and struggle with writing new content all the time.
Someone told me to use AI to do create articles which I am kinda considering because the output is almost written by human. Here is the sample content they sent me – https://sites.google.com/view/best-ai-content-writing-tools/home
Let me know if you think I should go ahead and use AI.
Great site you have here but I was wanting to know if you knew of any forums that cover the same topics discussed in this article? I’d really like to be a part of group where I can get advice from other knowledgeable individuals that share the same interest. If you have any suggestions, please let me know. Many thanks!