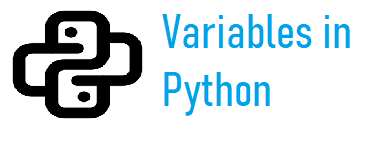
Variables in Python are declared using some set of rules as given below
- When you declare a variable in python then it must start with a letter or underscore character.
- You can not start with numbers or digit while declaring python variables.
- The variables consist of alphabets and numbers i.e. alphanumeric characters and underscore such as [A-Z, a-z, 0-9, _].
- There is no keyword or command for creating python variables like other programming languages. We can directly put the variable name such as x, y, etc.
- Python variables are case sensitive as ( phone, Phone, and PHONE) are three different variables.
Also read, Comments in Python 3
Below are some examples of variables in Python
Example 1:-
a = 5
b= 10
print(a)
print(b)
Output:-
5
10
Example 2:-
a = 5
a = 5.3
print(a)
Output:-
5.3
Example 3:-
name = Tom
Name = Dick
NAME = Herry
print(name)
print(Name)
print(NAME)
Output:-
File “d:\python\python_programming\01_python.py”, line 38, in
name = Tom
NameError: name ‘Tom’ is not defined
So, the correction of the above example 3 is as shown below
name = "Tom"
Name = "Dick"
NAME = "Herry"
print(name)
print(Name)
print(NAME)
Output:-
Tom
Dick
Herry
Also, we can display the multiple variables inside the print() function using a comma as shown below
Example 4:-
a = "Python is"
b = "high level"
c = "programming"
d = "language"
print(a,b,c,d)
Output:-Python is high level programming language
Also, we can display the above output with the help of the (+) operator as shown below
Example 5:-
a = "Python is"
b = "high level"
c = "programming"
d = "language"
print(a+b+c+d)
Output:- Python ishigh levelprogramminglanguage
You have noticed that the output does not contain the whitespaces. Follow the below example for the correct output.
a = "Python is "
b = "high level "
c = "programming "
d = "language"
print(a+b+c+d)
Output:- Python is high level programming language.
Now, the output is correct. So do not forget to include the spaces after each value of variables except the last variable.
Assign values to Python variables
We can assign more than one value in multiple variables as shown below
Example 6:-
a, b, c = "Book", "Pen", "Bags"
print(a)
print(b)
print(c)
Output:-Book
Pen
Bags
Also, we can assign a single value to multiple variables
Example 7:-
a=b=c="Book"
print(a)
print(b)
print(c)
Output:-Book
Book
Book
Unpacking of Python variables
Sometimes variables consist of multiple values and then we can assign that variable to multiple variables. Now, if you output the variables using the print() function then you can see, that each variable will produce the values of the first variable.
Example 8:-
cars = ["maruti","BMW","Toyota"]
a,b,c = cars
print(a)
print(b)
print(c)
Output:-
maruti
BMW
Toyota
Global variables in Python 3
When a variable is declared outside the function then it is considered a global variable. We can use this variable inside the function as shown below
Example 9:-
a = "High level programming language"
def myfunction():
print("Python is "+a)
myfunction()
Output:-
Python is High level programming language
Local variables in Python 3
When a variable is declared inside the function then it is considered a local variable. We can display the values of the local variable and global variable together as shown below
Example 10:-
a = "High level programming language"
def myfunction():
a = "Python is easy to learn"
print(a)
myfunction()
print("Python is "+a)
Output:-
Python is easy to learn
Python is High level programming language
Global keyword in Python 3
The global keyword is used to make a variable global which means we can change the local variable into global and we can access the local variable outside the function also.
Example 11:-
def myfunction():
global a
a = "Programming Language"
myfunction()
print("Python is a high level "+a)
Output:-
Python is a high level Programming Language
If you want to change the value of a global variable inside a function then you can do so with the help of a global keyword as shown below
Example 12:-
a = 10
def myfunction():
global a
a = 20
myfunction()
print("The value of a is ",a)
Output:-
The value of a is 20
Please note that we can concatenate strings and numbers together with the help of comma (,)
Also you can download the latest version of Python from here
Conclusion:- I hope this tutorial will help you to understand the overview of Python variables. If there is any doubt then please leave a comment below