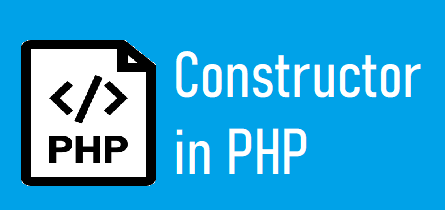
- Constructor in PHP is declared with __construct() function uing double underscore.
- When we define a class, we declare individual set methods to access the particular property of that class but if we initialize a constructor inside the parent class, then no need to include set methods.
- It helps us to reduce the number of set methods to declare for each public property of the class.
- PHP will automatically call the __construct() function as soon as the creation of the object of the parent class.
- It refers to the current object of your parent class.
- Also, we can create multiple objects of the parent class and access the properties passed inside the construct function with the help of each created object.
- We don’t need to initialize functions every time we declare public properties. Instead, we can use the constructor to do the same. So, the constructor is very userful in such cases.
- The constructor plays an important role in obeject-oriented programming. If you want to make your code easy and simple then you can use a constructor.
- We can pass the public properties of a class inside the __construct() function one by one as shown below.
Also read, OOPS concepts in PHP with real-time examples
With Constructor
<?php
class Car {
// Properties
public $name;
public $color;
function __construct($name,$color){
$this->name = $name;
$this->color = $color;
}
}
?>
Without Constructor
<?php
class Car {
// Properties
public $name;
public $color;
// Methods
function set_name($name) {
$this->name = $name;
}
function set_color($color){
$this->color = $color;
}
}
?>
Example to display the name and color of the car class
<!DOCTYPE html>
<html>
<body>
<?php
class Car {
// Properties
public $name;
public $color;
function __construct($name,$color){
$this->name = $name;
$this->color = $color;
}
// Methods
function get_name() {
return $this->name;
}
function get_color(){
return $this->color;
}
}
//create a new object
$first_car = new Car("Maruti","Red");
echo "The name of the car is: ".$first_car->get_name();
echo "<br>";
echo "The color of the car is: ".$first_car->get_color();
?>
</body>
</html>
Output:-The name of the car is: Maruti
The color of the car is: Red
If you want to run PHP in your local system then you can download the WAMP server from here.
Conclusion:- I hope this tutorial will help you to understand the overview of the constructor in PHP. If there is any doubt then please leave a comment below.