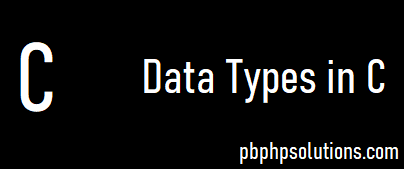
There are four basic data types in C language. They are given below along with the number of bytes occupied by them in the computer memory. (RAM)
Data Type | Bytes Occupied in RAM | Value Range | Value Range in Decimal |
char | 1 byte | -27 to 27-1 | -128 to 127 |
int | 2 bytes | -215 to 215 -1 | -32768 to 32767 |
float | 4 bytes | -231 to 231 -1 | 3.4e38 to 3.4e+38 |
double | 8 bytes | -263 to 263 -1 | 1.7e-308 to 1.7e+308 |
Note that the value range is calculated from the number of bits occupied by the data type. For example, int uses two bytes i.e. 16 bits; from this 1 bit is taken for sign (- or +). So, the range is expressed as -2 to the power 15 to 2 to the power 15-1. (Its decimal equivalent can be calculated by using a calculator but in the case of double, the calculator gives inaccurate answers since they are designed only to handle a small range of values.
Read also, Generation of Computers with their characteristics
The four basic data types in C language are defined as follows
- Char:- It refers to the character. It can hold one letter and symbol. In fact, the character in c is associated with integers to refer to a letter/symbol as per ASCII( American Standard Code for Information Interchange) that has assigned integer value for all letters/symbols used in programming.
- int:- This refers to an integer value. This is used to represent the signed or unsigned whole number within a specific range.
- float:- This refers to a floating-point or real number. It is used to represent a real number like 3.174813 or 4.52e6 with six decimal digits in decimal or exponential form.
- double:- This also refers to floating-point or real number. It can hold a real number in double precision. A double-precision number is used to represent 12 decimal digits like 3.412587632156 or 4.52145342148e12.
C language has additional data types along with the basic data types to enable the users to declare a variable in an appropriate type to improve the program execution speed and to refer to the largest and smallest numbers possible in programming for science and engineering applications.
Data Type | Bytes Occupied in RAM | Value Range | The range of values referred |
unsigned char | 1 byte | 0 to 28-1 | 0 to 255 |
short int | 1 byte | -27 to 27-1 | -128 to 127 |
unsigned short int | 1 byte | 0 to 28-1 | 0 to 255 |
unsigned int | 2 bytes | 0 to 216-1 | 0 to 65535 |
long int | 4 bytes | -231 to 231-1 | -2,147,483,648 to 2,147,483,647 |
unsigned long int | 4 bytes | 0 to 232-1 | 0 to 4,294,967,295 |
long double | 10 bytes | -279 to 279-1 | 3.4e-4932 to 1.1e+4932 |
Example of C data types
#include <stdio.h>
int main() {
// Create variables
int integerNumber = 10; // Integer number
float floatingNumber = 10.99; // Floating point number
char cahracterNumber = 'E'; // Character
// Print variables
printf("%d\n", integerNumber );
printf("%f\n", floatingNumber );
printf("%c\n", cahracterNumber );
return 0;
}
Output:-
10
10.99
E
Conclusion:- I hope this tutorial will help you to understand the overview of data types. If there is any doubt then please leave a comment below.