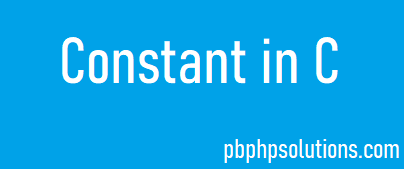
A constant in C is the unchanged value of a variable during the execution of the program. Suppose, if you declared an integer variable with a value of 10 as a constant variable and you want to change the value of that variable or assign a new value to that variable next time then you can not change it. If you change the value then it will throw an error during the execution.
A constant is declared with the help of the const keyword. Also, try to declare the constant variable with an uppercase letter.
Also read, Data Types in C with Examples
Let us take an example of a constant in C
#include <stdio.h>
int main() {
const int WHOLENUM = 15;
WHOLENUM = 10;
printf("%d", WHOLENUM);
return 0;
}
Explanation of the above code:-
- #include <stdio.h> is a header file library by which we can run input and output functions.
- Each program in C language starts with main() or int main() function. Inside this function, C program codes are executed and it starts with a curly brace and ends with a curly brace.
- printf() function is used to print or display the output or text on the screen after program execution.
Output:-

Types of Constant in C with Example
Numeric Constants:- There are two types of numeric constants.
- Integer Constant:- An integer constant is a signed or unsigned whole number. For eg:- -25, 350 etc.
- Real or Floating Point Constant:- Any signed or unsigned number with some fractional part is called a real or floating-point constant. It can be written in decimal or exponential form.
Example of floating-point constant in decimal form
0.254, +342
Example of floating-point constant in exponential form
0.218e6 means 0.218*106
String or Character Constant:- Any string of characters inside quotes is called a string constant or character constant. There are two types of string constants.
- Single character string constant:- Any letter or character enclosed inside a single quote is called a single character string constant. For eg:- ‘Y’, ‘S’
- String of characters constant:- Any string of characters including letters, digits, and symbols enclosed inside double quotes is called a string of characters constant. For eg:- “Total value is”
Conclusion:- I hope this tutorial will help you to understand the overview. If there is any doubt then please leave a comment below.