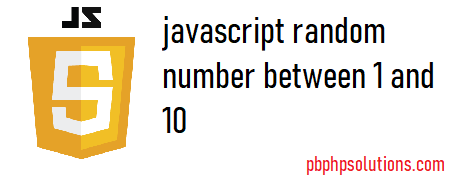
Hi friends, in this tutorial you will learn how to generate javascript random number between 1 and 10 which means you have to return all the numbers except 10 which means zero to nine. In order to generate random numbers, you should have the idea of two javascript functions as mentioned below
- Math.random()
- Math.floor()
Math.random( ):- This function always returns the numbers less than one which means it produces the numbers between zero and one or you can say including zero and excluding one.
Example:-
<html>
<head>
<title>Javascript random number example</title>
</head>
<body>
<h3>Below is a random number using Math.random()</h3>
<p id="random"></p>
<script type="text/javascript">
document.getElementById("random").innerHTML = Math.random();
</script>
</body>
</html>
Output:-
Below is a random number using Math.random()
0.7600114421439201
Math.floor( ) :- This function returns the number from the left side of a floating point number as shown in the example below
Example:-
<html>
<head>
<title>Javascript random number example</title>
</head>
<body>
<h3>Apply Math.floor() to 1.3</h3>
<p id="floor"></p>
<script type="text/javascript">
document.getElementById("floor").innerHTML = Math.floor(1.3);
</script>
</body>
</html>
Output:-
Apply Math.floor() to 1.3
1
Also read, Object in Javascript with example
Two ways to generate a javascript random number between 1 and 10
- Using Math.random() function with the help of Math.floor()
- Using javascript function with the help of minimum and maximum numbers.
Using Math.random() function with Math.floor()
If you want to generate a random number between 1 and 10 that means you are going to display the numbers from 0 to 9. In order to do so, you can use the Math.random() function with Math.floor() as shown in the example below
Example:-
<html>
<head>
<title>Javascript random number example</title>
</head>
<body>
<h3>Random number between 1 and 10</h3>
<p id="random_number"></p>
<script type="text/javascript">
document.getElementById("random_number").innerHTML = Math.floor(Math.random()*10);
</script>
</body>
</html>
Output:-
Random number between 1 and 10
4
Using javascript function with minimum and maximum number
As we all know that we can return any number or output inside the javascript function. Here, we will use a function using the formula as shown in the below example to return the numbers from 0 to 9.
Formula:- Math.floor(Math.random() * (max – min) ) + min;
Example:-
<html>
<head>
<title>Javascript random number example</title>
</head>
<body>
<button type="button" onclick="genearteRandomNumber()">Click here to generate random number between 1 and 10</button>
<p id="random_number_using_function"></p>
<script type="text/javascript">
function genearteRandomNumber()
{
document.getElementById("random_number_using_function").innerHTML = Math.floor(Math.random()*(10-1))+1;
}
</script>
</body>
</html>
Output:-

Conclusion:- I hope this tutorial will help you to understand the concept of producing a random number. If there is any doubt then please leave a comment below