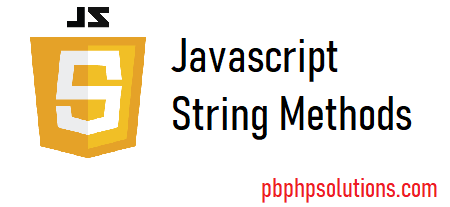
In this tutorial, you will learn the javascript string methods with examples one by one as given below
- length:- This property returns the length of the given string which means it counts every character of the string and returns the total number of characters in the string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph returns the length of the given string</p>
<p id="demo"></p>
<script>
let text = "Helloworld";
document.getElementById("demo").innerHTML = text.length;
</script>
</body>
</html>
Output:-
Below paragraph returns the length of the given string
10
Also read, Object in Javascript with example
slice():- This function extracts some part of the given string and returns the extracted string. This string method takes two parameters as the start position index and the end position index. Please note that the start position index starts from zero and the end position index can not take place in the extracted string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph extracts a part of the string and returns the extracted string</p>
<p id="demo1"></p>
<script>
let text = "Helloworld";
document.getElementById("demo1").innerHTML = text.slice(0,5);
</script>
</body>
</html>
Output:-
Below paragraph extracts a part of the string and returns the extracted string
Hello
substring():- This function is also as same as the slice() function and returns the extracted string from the given string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph extracts a part of the string and returns the extracted string</p>
<p id="demo2"></p>
<script>
let text = "Helloworld";
document.getElementById("demo2").innerHTML = text.substring(0,5);
</script>
</body>
</html>
Output:-
Below paragraph extracts a part of the string and returns the extracted string
Hello
substr( ):- This function is also similar to the substring() function and takes exactly two parameters and returns the extracted string from the given string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph extracts a part of the string and returns the extracted string</p>
<p id="demo3"></p>
<script>
let text = "Helloworld";
document.getElementById("demo3").innerHTML = text.substr(0,5);
</script>
</body>
</html>
Output:-
Below paragraph extracts a part of the string and returns the extracted string
Hello
replace():- This function replaces some part of the string with a new string inside the given string. This function takes exactly two parameters as given below
- The first parameter defines the text to be replaced
- The second parameter defines the replaced text
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph replaces a part of the string with a new string</p>
<p id="demo4"></p>
<script>
let text = "Helloworld";
document.getElementById("demo4").innerHTML = text.replace("world","India");
</script>
</body>
</html>
Output:-
Below paragraph replaces a part of the string with a new string
HelloIndia
replace( ) with g:- This function replaces all the matching parts of the given string with a new string. This function takes exactly two parameters as given below
- The first parameter defines all the matching text to be replaced.
- The second parameter defines the replaced text.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph replaces all the matching parts of the string with a new string</p>
<p id="demo5"></p>
<script>
let string = "If you want to visit Inida then you should visit the capital of India";
document.getElementById("demo5").innerHTML = string.replace(/you/g,"I");
</script>
</body>
</html>
Output:-
Below paragraph replaces all the matching parts of the string with a new string
If I want to visit India then I should visit the capital of India
toUpperCase( ):- This function converts all the characters of the given string into uppercase. This function does not require any parameter.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph makes the given string in uppercase.</p>
<p id="demo6"></p>
<script>
let string = "If you want to visit Inida then you should visit the capital of India";
document.getElementById("demo6").innerHTML = string.toUpperCase();
</script>
</body>
</html>
Output:-
Below paragraph makes the given string in uppercase.
IF YOU WANT TO VISIT INIDA THEN YOU SHOULD VISIT THE CAPITAL OF INDIA
toLowerCase():- This function converts all the characters of the given string into lowercase. This function also does not require any parameter.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph makes the given string in lowercase.</p>
<p id="demo7"></p>
<script>
let string = "If you want to visit Inida then you should visit the capital of India";
document.getElementById("demo7").innerHTML = string.toLowerCase();
</script>
</body>
</html>
Output:-
Below paragraph makes the given string in lowercase.
if you want to visit inida then you should visit the capital of india
trim( ):- This function removes or eliminates all the whitespaces found in the given string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph removes whitespaces from the both side of a string</p>
<p id="demo9"></p>
<script>
let trimstring = "Helloworld";
document.getElementById("demo9").innerHTML = trimstring.trim();
</script>
</body>
</html>
Output:-
Below paragraph removes whitespaces from the both side of a string
Helloworld
charAt():- This function will return the searched character from the given string. Suppose we want to search for a particular character from the given string then this function will help us to find that character in the given string. It starts counting the character from position zero.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph will diplay the searched character from a string</p>
<p id="demo10"></p>
<script>
let text = "Helloworld";
document.getElementById("demo10").innerHTML = text.charAt(5);
</script>
</body>
</html>
Output:-
Below paragraph will diplay the searched character from a string
w
charCodeAt():- This function will return the Unicode number of characters from the given string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph will diplay the unicode character from a string</p>
<p id="demo11"></p>
<script>
let text = "Helloworld";
document.getElementById("demo11").innerHTML = text.charCodeAt(5);
</script>
</body>
</html>
Output:-
Below paragraph will diplay the unicode character from a string
119
padStart():- This function appends a new string at the start of the given string. This function takes exactly two parameters as given below
- The first parameter defines the number of times the new string is to be added
- The second parameter defines the new string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph will pads a string at the start with another string</p>
<p id="demo12"></p>
<script>
let padstring = 3;
let padnumberstring = padstring.toString();
document.getElementById("demo12").innerHTML = padnumberstring.padStart(5,'y');
</script>
</body>
</html>
Output:-
Below paragraph will pads a string at the start with another string
yyyy3
padEnd():- This function appends a new string at the end of the given string. This function takes exactly two parameters as given below
- The first parameter defines the number of times the new string is to be added.
- The second parameter defines the new string.
Note:- If the given string is a number then you should convert it to a string first using the toString() function in both the padStart() and padEnd() methods.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph will pads a string at the end with another string</p>
<p id="demo13"></p>
<script>
let padstring = 3;
let padnumberstring = padstring.toString();
document.getElementById("demo13").innerHTML = padnumberstring.padEnd(5,'y');
</script>
</body>
</html>
Output:-
Below paragraph will pad a string at the end with another string
3yyyy
concat():- This method appends a new string with the given string.
Example:-
<html>
<head>
<title>Javascript string methods</title>
</head>
<body>
<p>Below paragraph will join two strings together</p>
<p id="demo14"></p>
<script>
let text1 = "Hello";
let text2 = "World";
document.getElementById("demo14").innerHTML = text1.concat(" ",text2);
</script>
</body>
</html>
Output:-
Below paragraph will join two strings together
Hello World
Conclusion: I Hope this tutorial will help you to understand the concept of javascript string methods. If there is any doubt then please leave a comment below.