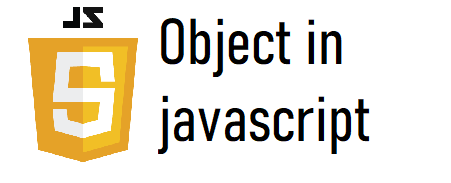
- Object in javascript is a kind of container for storing values or you can say it is a kind of variable with multiple values. As we all know that a javascript variable contains a single value which means we can only assign a single value to a javascript variable.
- It holds the values using name: value pair.
- javascript object is declared with the keyword const.
- parenthesis is used to declare javascript objects.
Syntax:-const object = {name:value,name1:value1,name2:value2};
Below is an example of a javascript object
const boy = {first_name: "Robin",last_name: "Sinha",age:30};
Also, you can write the above object as shown belowconst boy = {
first_name: "Robin",
last_name: "Sinha",
age: 30
};
whereas boy is the name of the object
first_name is the property name
Robin is the property value
From the above example, you can see some values inside the object. Those values are known as object properties. These values can be used in the methods if we declare the methods along with the object properties. Also, note that we have to use the first bracket at the time of calling methods using the javascript objects.
If you do not call the method using the first bracket then it will return the function definition declared inside the object instead of returning the result of the defined methods. On the other hand, you can access the properties or methods of an object with the help of an HTML id attribute.
Also read, Google Maps Add Marker Using Maps Javascript API and HTML
Access the properties of an object in javascript
We can access the properties of a javascript object in two ways as given below
first way:- object.property_name that means boy.first_name
second way:- object[“property_name”] that means boy[“property_name”]
Below is an example
<html>
<head>
<title>javascript example</title>
</head>
<body>
<p>Below paragraph includes the name and age of the boy</p>
<p id="obj" style="font-weight: bolder;"></p>
<script>
const boy = {
first_name: "Robin",
last_name: "Sinha",
age: 23,
};
document.getElementById("obj").innerHTML = "The name of the boy is "+boy.first_name+" and age is "+boy.age;
</script>
</body>
</html>
Output:-
Below paragraph includes the name and age of the boy
The name of the boy is Robin and age is 23
Object methods:-
Object method is a kind of property inside the javascript object and it is stored in object properties with the help of function definition.
Below is an example
<html>
<head>
<title>Javascript example</title>
</head>
<body>
<p>Full name of the boy is</p>
<p id="fullname"></p>
<script>
const boy = {
first_name: "Robin",
last_name: "Sinha",
age: 23,
full_name: function(){
return this.first_name+" "+this.last_name;
}
};
document.getElementById("fullname").innerHTML = boy.full_name();
</script>
</body>
</html>
Output:-
Full name of the boy is
Robin Sinha
Conclusion:- I hope this tutorial will help you to understand the concept. If there is any doubt then please leave a comment below