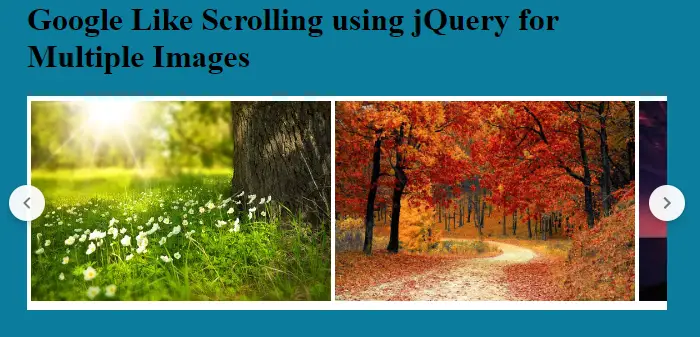
In this tutorial, you will learn how to use Google like scrolling using jQuery for multiple images. Sometimes we need to scroll the gallery images horizontally one after another. We can do so with the help of HTML and CSS or by using various jQuery plugins available online. But here, I will discuss a g-scrolling carousel plugin that is very easy to integrate.
Also read, Javascript String Methods with example
Required steps for Google like scrolling using jQuery
Step 1:- First of all, you have to download the plugin from here.
Step 2:- Create an HTML file and include some images inside the below divs as shown below.
<div class="container">
<h1>Google Like Scrolling using jQuery for Multiple Images</h1>
<div class="g-scrolling-carousel">
<div class="items">
<img src="nature1.jpg" width="300" height="200">
<img src="nature2.jpg" width="300" height="200">
<img src="nature3.jpg" width="300" height="200">
<img src="nature4.jpg" width="300" height="200">
</div>
</div>
</div>
Step 3:- Extract the plugin zip folder and place the extracted folder along with the HTML file.
Step 4:- Include the CSS link in your HTML file as shown below
<link href="google-scrolling-carousel/jquery.gScrollingCarousel.css" rel="stylesheet" />
Step 5:- Add some custom styles using CSS inside the head section of the HTML file as shown below.
<style type="text/css">
body{
background-color: #0C7C9C;
}
.container { margin: 150px auto; max-width: 640px; }
.g-scrolling-carousel .items{
padding: 5px 0;
background-color: #fff;
}
.g-scrolling-carousel .items a {
display: inline-block; /* notice the comments between inline-block items */
margin-right: 10px;
width: 300px;
height: 250px;
line-height: 250px;
box-shadow: 0 3px 6px rgba(0, 0, 0, 0.16), 0 3px 6px rgba(0, 0, 0, 0.23);
text-align: center;
text-decoration: none;
}
</style>
Step 5:- Include the javascript links in your HTML file before the body tag as shown below
<script src="https://code.jquery.com/jquery-3.4.1.js"></script>
<script src="google-scrolling-carousel/jquery.gScrollingCarousel.js"></script>
Step 6:- Include the jQuery piece of code as shown below
<script type="text/javascript">
$(function(){
$(".g-scrolling-carousel .items").gScrollingCarousel();
});
</script>
Explanation:-
In step 2, you have seen that I have used four images and all are wrapped inside two divs with items class and g-scrolling-carousel respectively. By using these two classes gScrollingCarousel() function is called for which the images are sliding horizontally. Please do not forget to wrap the images inside the items div otherwise the scrolling will not work.
Complete Code:-
<!DOCTYPE html>
<html>
<head>
<title></title>
<link href="google-scrolling-carousel/jquery.gScrollingCarousel.css" rel="stylesheet" />
<style type="text/css">
body{
background-color: #0C7C9C;
}
.container { margin: 150px auto; max-width: 640px; }
.g-scrolling-carousel .items{
padding: 5px 0;
background-color: #fff;
}
.g-scrolling-carousel .items a {
display: inline-block; /* notice the comments between inline-block items */
margin-right: 10px;
width: 300px;
height: 250px;
line-height: 250px;
box-shadow: 0 3px 6px rgba(0, 0, 0, 0.16), 0 3px 6px rgba(0, 0, 0, 0.23);
text-align: center;
text-decoration: none;
}
</style>
</head>
<body>
<div class="container">
<h1>Google Like Scrolling using jQuery for Multiple Images</h1>
<div class="g-scrolling-carousel">
<div class="items">
<img src="nature1.jpg" width="300" height="200">
<img src="nature2.jpg" width="300" height="200">
<img src="nature3.jpg" width="300" height="200">
<img src="nature4.jpg" width="300" height="200">
</div>
</div>
</div>
<script src="https://code.jquery.com/jquery-3.4.1.js"></script>
<script src="google-scrolling-carousel/jquery.gScrollingCarousel.js"></script>
<script type="text/javascript">
$(function(){
$(".g-scrolling-carousel .items").gScrollingCarousel();
});
</script>
</body>
</html>
Conclusion:- I hope this tutorial will help you to understand the concept. If there is any doubt then please leave a comment below.