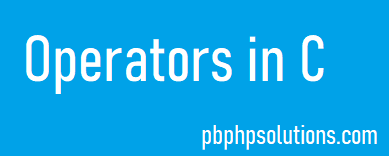
In this tutorial, you will learn the operators in c with examples one by one. C language uses different types of operators as given below
- Arithmetic operators
- Relational operators
- Logical operators
- Increment and decrement operators
- Assignment operators
- Conditional operator
- Bitwise operators
Arithmetic Operators:- Arithmetic operators are used to perform various kinds of arithmetic operations.
Also read, constant in C with example
Arithmetic operators used in C language are given below
Operator | Meaning | Example | Result |
+ | addition | 5+2 | 7 |
– | subtraction | 5-2 | 3 |
* | multiplication | 5*2 | 10 |
/ | division | 5/2 | 2 |
% | modulus operator to get remainder in integer division | 5%2 | 1 |
Note that when the two operands (5 and 2) are integers, the result will be an integer. To obtain the real value or a floating-point number in the result, at least one of the operands must be float or double.
Below is an example to find float or real value
5.0/2.0 or
5/2.0 or
5.0/2 will produce the result of 2.5
Below is an example to find the remainder of modulus operations
7 % 4 will produce the result 3
An expression consisting of numeric variables, constants, and arithmetic operators is called arithmetic expression. The value produced by evaluating an arithmetic expression will always be numeric i.e. integer, float or double, etc.
Relational operators:- Relational operators are used to compare the values of operands or expressions to obtain the result in a boolean value i.e. true or false.
Relational operator used in C language are given below
Operator | Meaning | Example | Result |
< | less than | 5<2 | false |
> | greater than | 5>2 | true |
<= | less than or equal to | 5<=2 | false |
>= | greater than or equal to | 5>=2 | true |
== | equal to | 5==2 | false |
!= | not equal to | 5!=2 | true |
Note that the boolean value “true” is represented by 1 in C language and false is represented by 0.
Logical operators:- Logical operators are used to combining more relational operations to form a complex expression called logical expression. A value obtained by evaluating a logical expression will always be logical i.e. either true or false.
Logical operator used in C language are given below
Operator | Meaning | Example | Result |
&& | logical and | (5<2) && (5>3) | false |
|| | logical or | (5<2) || (5>3) | true |
! | logical not | !(5<2) | true |
Note that the logical not(!) is a unary operator which requires only one operand. it is also referred to as an inverter that converts the value of operands from true to false and vice versa.
Increment and Decrement operators(++ and –):- Increment operators are used to increase the value of an integer variable by 1. The decrement operator is used to deduct the value of an integer variable by 1.
Consider the following example
m=15;
m++ or ++m will produce the same result m=16
m– or –m will produce the same result m=14;
Note that m++ or m– are referring to the postfix increment and decrement operation and ++m or –m are referring to the prefix increment and decrement operation.
Consider the following example
m=15;
k = m++; // assigns the value 15 to k and increases the value of m to 16 thereafter.
m=15;
k = ++m; // increases the value of m to 16 and then assigns 16 to k;
Assignment Operators:- Assignment operators are used to perform arithmetic operations while assigning a value to a variable.
Assignment operators used in C language are given below
Operator | Example | Equivalent Expression | Result |
+= | m+=10 | m = m+10 | 25 |
-= | m -= 10 | m = m-10 | 5 |
*= | m*=10 | m = m*10 | 150 |
/= | m/=10 | m = m/10 | 1 |
%= | m%=10 | m = m%10 | 5 |
Conditional Operator (?:) or Ternary Operator:-
A conditional operator is used to check a condition and select a value depending on the value of the condition. Normally, the selected value will be assigned to a variable that has the following formvariable = (condition)? value 1:value 2;
when this operator is executed by the computer, the value of the condition is evaluated. If it is true then value 1 is assigned to the variable, otherwise, value 2 is assigned to the variable.
Consider the following example
big = (a>b) ? a:b
In this operation, the computer checks the value of the condition (a>b); if it is true ‘a’ is assigned to big; otherwise, b is assigned to big. This is similar to the usual method of writing a condition which is shown below
if(a>b)
big = a;
else
big = b;
Bitwise operators:- Bitwise operators are used to perform operations at the binary digit level. These operators are not commonly used and are used only in special applications where optimized use of storage is required.
Bitwise operators used in C language are given below
Operator | Meaning |
<< | Shifts the bits to the left |
>> | Shifts the bits to the right |
~ | bitwise inversion |
& | bitwise logical and |
| | | bitwise logical or |
^ | bitwise exclusive or |
Additional Operators used in C language
There are another two operations that are used in the c program.
- sizeof operator.
- comma operator.
sizeof operator:- sizeof operator is used to finding the number of bytes occupied by a variable or data type in the computer memory.
Some examples are given below
int m, x[50];
declares an array of 50 elements and a variable m.
sizeof(float);
returns 4 which is the number of bytes occupied by float type data.
sizeof(m);
returns 2 which is the number of bytes occupied by the integer variable m.
sizeof(x);
returns 100 which is the number of bytes occupied by the array x.
Comma Operator:- The comma operator is used to linking related expressions to make the program more compact. Related expressions are evaluated from left to right and the value of the right most expression is returned as the value of the expression.
Consider the below exampletemp = x;
x = y;
y = temp;
The above lines can be written as a single expression with the help of a comma operator as shown belowtemp = x,x = y, y = temp;
Conclusion:- I hope this tutorial will help you to understand the overview of operators in C. If there is any doubt then please leave a comment below.