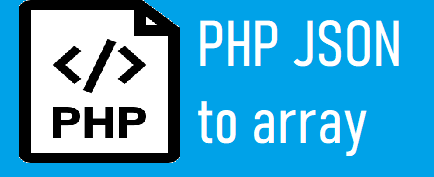
In this tutorial, you will learn how to decode PHP JSON to array with a detailed explanation. This is a very important feature often used in web development but before getting started, we must know about JSON as mentioned below
What is JSON
JSON is a javascript object notation and text-based format that is used to store data and exchange those data when needed. With the help of JSON, we can send data to the server and get the response from the server in JSON format. JSON can be used in any programming language.
Syntax:
{'object key':object value,'object key1':object value1,'object key2':object value2}
json_decode() function
- This is a built-in PHP function that is used to convert the JSON data into a PHP object or an associative array.
- This function has a second parameter and if this parameter is set to true then it will return the JSON data into an associative array.
Also read, OOPS concepts in PHP with realtime examples
Below are some of the examples of json_decode
Example 1: to convert the JSON data into a PHP object
<?php
$brothers = '{"Ramen":40,"Robin":37,"Ramesh":31}';
$brothersobj = json_decode($brothers);
print_r($brothersobj);
?>
Output:-
stdClass Object ( [Ramen] => 40 [Robin] => 37 [Ramesh] => 31 )
Example 2: to convert the JSON data into an associative array
<?php
$brothers = '{"Ramen":40,"Robin":37,"Ramesh":31}';
$brothersobj = json_decode($brothers,true);
print_r($brothersobj);
?>
Output:-
Array ( [Ramen] => 40 [Robin] => 37 [Ramesh] => 31 )
How to get the values of PHP object from the JSON data
<?php
$brothers = '{"Ramen":40,"Robin":37,"Ramesh":31}';
$brothersobj = json_decode($brothers);
//print_r($brothersobj);
//Access the decoded values one by one from a PHP object
echo "The value of the first object is: ".$brothersobj->Ramen;
echo "<br>";
echo "The value of the second object is: ".$brothersobj->Robin;
echo "<br>";
echo "The value of the third object is: ".$brothersobj->Ramesh;
?>
Output:-
The value of the first object is: 40
The value of the second object is: 37
The value of the third object is: 31
How to get the values of associative array from the JSON data
<?php
$brothers = '{"Ramen":40,"Robin":37,"Ramesh":31}';
$brothersobj = json_decode($brothers,true);
//print_r($brothersobj);
//Access the decoded values one by one from an associative array
echo "The value of the first object is: ".$brothersobj['Ramen'];
echo "<br>";
echo "The value of the second object is: ".$brothersobj['Robin'];
echo "<br>";
echo "The value of the third object is: ".$brothersobj['Ramesh'];
?>
Output:-
The value of the first object is: 40
The value of the second object is: 37
The value of the third object is: 31
Get the values of PHP object using the foreach loop
<?php
$brothers = '{"Ramen":40,"Robin":37,"Ramesh":31}';
$brothersobj = json_decode($brothers);
//print_r($brothersobj);
echo "<br>";
//Get the values of the object using foreach loop
foreach ($brothersobj as $key => $value) {
// code...
echo $key .' => '.$value.'<br>';
}
?>
Output:-Ramen => 40
Robin => 37
Ramesh => 31
Get the values of the associative array using the foreach loop
<?php
$brothers = '{"Ramen":40,"Robin":37,"Ramesh":31}';
$brothersobj = json_decode($brothers,true);
echo "<br>";
//Get the values of the object using foreach loop
foreach ($brothersobj as $key => $value) {
// code...
echo $key . '=> '.$value.'<br>';
}
?>
Output:-Ramen=> 40
Robin=> 37
Ramesh=> 31
Also read, How to display data from database in php
Conclusion:- I hope this tutorial will help you to understand the concept. If there is any doubt then please leave a comment below