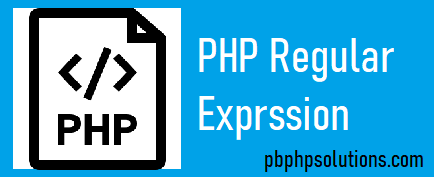
- PHP Regular Expression is a kind of pattern that consists of a single character or a combination of more than one character.
- It is used to search for a specific character or a combination of characters inside a string.
- It is also used to replace some characters or some words inside a string.
- It is also used to replace a string with another string.
Syntex:-$reg_exp = "/pattern/i";
- whereas ‘/’ is the delimiter that is used to start the regular expression. This can be any character except a letter, number, backslash or spaces, etc. Sometimes if your pattern contains a forward slash then you can choose other special characters such as @#~ etc instead of forwardslash(/) as a delimiter.
- The ‘pattern’ is the query string that needs to be searched inside another string.
- ‘i’ is used to perform the search operation case sensitive. Suppose if you have the search pattern that is found in the main string with similar characters whether it may be in uppercase or lowercase or mixed case then the regular expression will be executed and it will return the response as 1. If your search pattern does not match the characters with the main string then the regular expression will not be executed and you will get the response as 0.
Also read, PHP JSON to Array with Example
Some important PHP regular expression functions
preg_match():- This function is used to match a pattern in a string whether the pattern is found or not inside that string.
Below are some examples of preg_match()
Example 1:-
<?php
$str = "Regular expression is used to perform text based search operations";
$pattern = "/ion/i";
echo preg_match($pattern, $str);
?>
Output:- 1
In the above example, the output is 1 which means the pattern you have searched for is found
Example 2:-
<?php
$str = "Regular expression is used to perform text based search operations";
$pattern = "/ixz/i";
echo preg_match($pattern, $str);
?>
Output:- 0
In the above example, the output is 0 which means the pattern you have searched for is not found.
preg_match_all():- This function is used to count the total number of matches of your search pattern inside the main string.
Below is an example of preg_match_all()
<?php
$str = "Regular expression is used to perform text based search operations";
$pattern = "/ion/i";
echo preg_match_all($pattern, $str);
?>
Output:- 2
In the above example, the output is 2 which means your search pattern is found two times inside the main string.
preg_replace():- This function is used to replace a text or combination of characters or a combination of words with a new string or combination of characters inside the main string.
Below is an example of preg_replace():-
<?php
$str = "Regular expression is used to perform text based search operations";
$pattern = "/perform text based search operations/i";
echo preg_replace($pattern, 'find sequence of characters inside a string', $str);
?>
Output:-
Regular expression is used to find sequence of characters inside a string
Also read, How to display data from database in php
You can download the XAMPP server to run php programs locally.
Conclusion:- I hope this tutorial will help you to understand the concept of regular expression. If there is any doubt then please leave a comment below