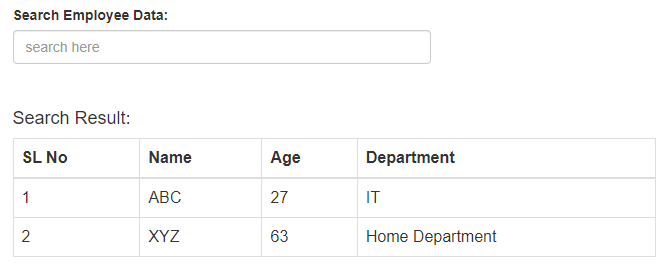
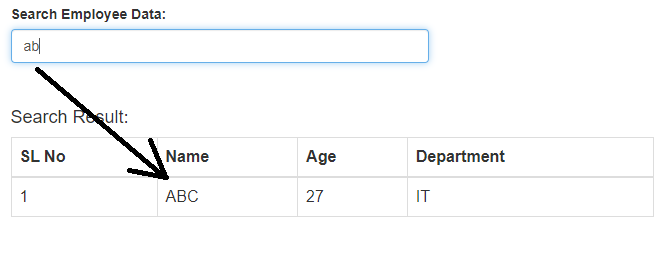
In this tutorial, we will learn Laravel 8 autocomplete search from the database using ajax and Jquery.
The autocomplete search is very helpful because we can search the data from the database just by typing the information we want instead of reloading the page. Also, it is a very important part of a good UI of the application.
We are able to do the autocomplete search because of AJAX and Jquery. Without AJAX, we can not search the data on the fly i.e. without submitting any search button.
Required steps of Laravel 8 autocomplete search from database
Step 1:- Create a project in laravel 8
Please Read, How to create laravel project from scratch step by step
Step 2:- Set up the database.
DB_CONNECTION=mysql
DB_HOST=127.0.0.1
DB_PORT=3306
DB_DATABASE=laravel
DB_USERNAME=root
DB_PASSWORD=
Step 3:- Create a table in the database
CREATE TABLE `employee` (
`id` int(10) unsigned NOT NULL AUTO_INCREMENT,
`name` varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`age` varchar(20) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`department` varchar(255) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`created_at` timestamp NOT NULL DEFAULT current_timestamp() ON UPDATE current_timestamp(),
`updated_at` timestamp NOT NULL DEFAULT '0000-00-00 00:00:00',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=3 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
Step 4:- Set up routes in the web.php file inside the routes folder as shown below
Route::get('/searchdata',[App\Http\Controllers\EmployeeController::class, 'index']);
Route::get('/empsearch',[App\Http\Controllers\EmployeeController::class, 'searchemp']);
We can see from the above routes that the EmployeeController has two methods
- index()
- searchemp()
The index() method is used for viewing the employee information from the database in the blade file.
On the other hand, the searchemp() method is used to search the required information from the database based on the user request and return the response data to the user.
Step 5:- Create a model using the artisan command in the command terminal as shown below
php artisan make:model Employee
The above command will create a model like app\Models\Employee.php
Employee.php:-
<?php
namespace App\Models;
use Illuminate\Database\Eloquent\Factories\HasFactory;
use Illuminate\Database\Eloquent\Model;
class Employee extends Model
{
use HasFactory;
protected $table = 'employee';
protected $fillable = [
'name','age','department',
];
}
Step 6:- Create a controller using the artisan command in the command terminal as shown below
php artisan make:controller EmployeeController
EmployeeController.php:-
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use App\Models\Employee;
use DB;
use Auth;
class EmployeeController extends Controller
{
//
public function index(){
$data = Employee::all();
return view('searchdata',compact('data'));
}
public function searchemp(Request $request){
$searchvalue = $request->search;
if($request->ajax()){
$empinfo = DB::table('employee')
->where('name','LIKE','%'.$searchvalue.'%')
->get();
if (count($empinfo)>0) {
$output = '';
foreach ($empinfo as $key=>$value){
$output .= '<tr>
<td>'.($key+1).'</td>
<td>'.($value->name).'</td>
<td>'.$value->age.'</td>
<td>'.$value->department.'</td>
</tr>';
}
}
else {
$empinfo = Employee::all();
$output = '';
foreach ($empinfo as $key=>$value){
$output .= '<tr>
<td>'.($key+1).'</td>
<td>'.($value->name).'</td>
<td>'.$value->age.'</td>
<td>'.$value->department.'</td>
</tr>';
}
}
return $output;
}
}
}
Step 7:- Create a blade file inside the resources\views folder of the laravel project.
searchdata.blade.php:-
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/css/bootstrap.min.css">
<style>
.container{
width:50%;
height:30%;
padding:20px;
}
</style>
</head>
<body>
<div class="container">
<br/><br/>
<div class="row">
<div class="col-sm-8">
<div class="form-group">
<label for="name">Search Employee Data:</label>
<input type="search" class="form-control" onkeyup="fetchDataEmp()" id="find" placeholder="search here">
</div>
</div>
</div>
<br/>
<h4>Search Result:</h4>
<table class="table table-bordered">
<thead>
<tr>
<th>SL No</th>
<th>Name</th>
<th>Age</th>
<th>Department</th>
</tr>
</thead>
<tbody>
@foreach($data as $key=>$value)
<tr>
<td>{{$key+1}}</td>
<td>{{$value->name}}</td>
<td>{{$value->age}}</td>
<td>{{$value->department}}</td>
</tr>
@endforeach
</tbody>
</table>
<script src="https://ajax.googleapis.com/ajax/libs/jquery/3.5.1/jquery.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.4.1/js/bootstrap.min.js"></script>
<script>
function fetchDataEmp(){
var search = $('#find').val();
//alert(search);
$.ajax({
url: 'empsearch',
type: 'get',
data: {'search':search},
success:function(data){
$('tbody').html(data);
}
});
}
</script>
</body>
</html>
Step 8:- Now, open the terminal and type the command as shown below
php artisan serve
The above command will generate the link as shown below
http://127.0.0.1:8000
Step 9:- Now, open the browser and hit the below URL
http://127.0.0.1:8000/searchdata
Conclusion:- I hope this tutorial will help you to understand the overview. If you have any doubt then please leave a comment below.